Springboot整合Ehcache 解决Mybatis二级缓存数据脏读 -详细
时间: 2024-03-05 11:48:58 浏览: 213
好的,下面是Spring Boot整合Ehcache解决Mybatis二级缓存数据脏读的详细步骤:
1. 添加依赖
首先,需要在pom.xml文件中添加Ehcache和Spring Boot的相关依赖:
```
<!-- Ehcache依赖 -->
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>2.10.6</version>
</dependency>
<!-- Spring Boot依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.3</version>
</dependency>
```
2. 配置Ehcache
在src/main/resources目录下创建ehcache.xml文件,并添加以下配置:
```
<?xml version="1.0" encoding="UTF-8"?>
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://ehcache.org/ehcache.xsd"
updateCheck="false">
<!-- 默认缓存配置 -->
<defaultCache
maxEntriesLocalHeap="10000"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"
diskSpoolBufferSizeMB="30"
maxEntriesLocalDisk="10000000"
diskExpiryThreadIntervalSeconds="120"
memoryStoreEvictionPolicy="LRU"
statistics="true">
</defaultCache>
<!-- Mybatis二级缓存配置 -->
<cache name="com.example.demo.mapper.UserMapper"
maxEntriesLocalHeap="10000"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"
diskSpoolBufferSizeMB="30"
maxEntriesLocalDisk="10000000"
diskExpiryThreadIntervalSeconds="120"
memoryStoreEvictionPolicy="LRU"
statistics="true">
</cache>
</ehcache>
```
在配置文件中,我们定义了默认缓存配置和针对UserMapper的缓存配置。
3. 配置Mybatis
在application.properties中添加Mybatis相关配置:
```
# Mybatis配置
mybatis.type-aliases-package=com.example.demo.entity
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.configuration.cache-enabled=true
mybatis.configuration.local-cache-scope=session
mybatis.configuration.cache-ref=ehcache
```
注意,cache-enabled属性设置为true,local-cache-scope属性设置为session,并指定了使用的缓存提供者为Ehcache。
4. 配置缓存管理器
在启动类中配置缓存管理器:
```
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
EhCacheCacheManager cacheManager = new EhCacheCacheManager();
cacheManager.setCacheManager(ehCacheManagerFactoryBean().getObject());
return cacheManager;
}
@Bean
public EhCacheManagerFactoryBean ehCacheManagerFactoryBean() {
EhCacheManagerFactoryBean cacheManagerFactoryBean = new EhCacheManagerFactoryBean();
cacheManagerFactoryBean.setConfigLocation(new ClassPathResource("ehcache.xml"));
cacheManagerFactoryBean.setShared(true);
return cacheManagerFactoryBean;
}
}
```
在这里,我们通过EhCacheCacheManager和EhCacheManagerFactoryBean来配置缓存管理器。
5. 开启缓存
最后,在Mapper接口中开启缓存:
```
@CacheNamespace(implementation = net.sf.ehcache.Cache.class, eviction = FifoPolicy.class)
public interface UserMapper {
@Select("SELECT * FROM user WHERE id = #{id}")
@Options(useCache = true, flushCache = Options.FlushCachePolicy.FALSE, timeout = 10000)
User selectById(Integer id);
@Update("UPDATE user SET name = #{name} WHERE id = #{id}")
void updateNameById(@Param("id") Integer id, @Param("name") String name);
}
```
使用@CacheNamespace注解来指定缓存实现类和缓存策略,在每个查询方法上使用@Options注解来开启缓存。
至此,Spring Boot整合Ehcache解决Mybatis二级缓存数据脏读的配置就完成了。
阅读全文
相关推荐
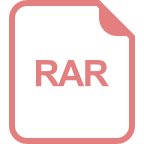
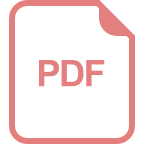

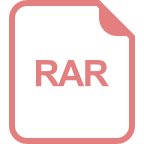
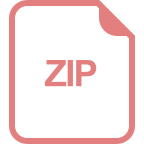
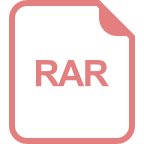
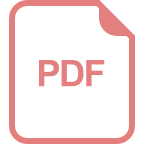
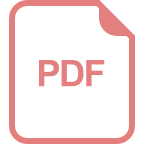
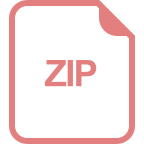
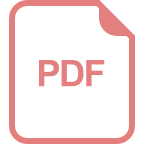
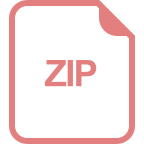
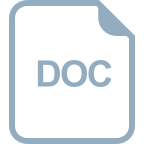
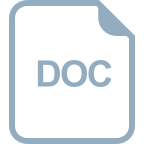
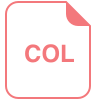
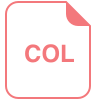
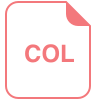
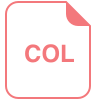