用java代码写一个应用程序,实现输入一个文件夹目录,或者文件目录,把整个文件夹(包括里面的子文件夹)压缩成rar或zip文件,也可以实现rar或zip文件的解压缩
时间: 2023-04-10 21:00:43 浏览: 126
你好,这个问题可以回答。以下是Java代码示例:
压缩文件夹:
```java
import java.io.*;
import java.util.zip.*;
public class ZipFolder {
public static void main(String[] args) throws Exception {
String sourceFolder = "path/to/source/folder";
String zipFile = "path/to/zip/file.zip";
zipFolder(sourceFolder, zipFile);
}
public static void zipFolder(String sourceFolder, String zipFile) throws Exception {
FileOutputStream fos = new FileOutputStream(zipFile);
ZipOutputStream zos = new ZipOutputStream(fos);
addFolderToZip("", sourceFolder, zos);
zos.close();
fos.close();
}
private static void addFolderToZip(String path, String sourceFolder, ZipOutputStream zos) throws Exception {
File folder = new File(sourceFolder);
for (String fileName : folder.list()) {
String filePath = sourceFolder + File.separator + fileName;
if (new File(filePath).isDirectory()) {
addFolderToZip(path + folder.getName() + "/", filePath, zos);
} else {
byte[] buffer = new byte[1024];
FileInputStream fis = new FileInputStream(filePath);
zos.putNextEntry(new ZipEntry(path + folder.getName() + "/" + fileName));
int length;
while ((length = fis.read(buffer)) > 0) {
zos.write(buffer, 0, length);
}
zos.closeEntry();
fis.close();
}
}
}
}
```
解压缩文件:
```java
import java.io.*;
import java.util.zip.*;
public class UnzipFile {
public static void main(String[] args) throws Exception {
String zipFile = "path/to/zip/file.zip";
String destFolder = "path/to/destination/folder";
unzipFile(zipFile, destFolder);
}
public static void unzipFile(String zipFile, String destFolder) throws Exception {
byte[] buffer = new byte[1024];
ZipInputStream zis = new ZipInputStream(new FileInputStream(zipFile));
ZipEntry zipEntry = zis.getNextEntry();
while (zipEntry != null) {
String fileName = zipEntry.getName();
File newFile = new File(destFolder + File.separator + fileName);
new File(newFile.getParent()).mkdirs();
FileOutputStream fos = new FileOutputStream(newFile);
int length;
while ((length = zis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fos.close();
zipEntry = zis.getNextEntry();
}
zis.closeEntry();
zis.close();
}
}
```
阅读全文
相关推荐
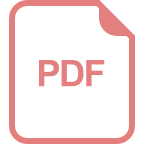

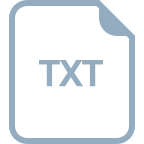
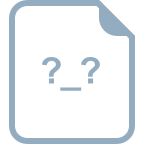
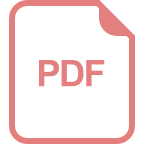
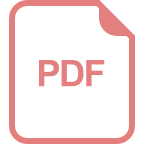
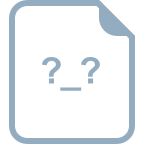
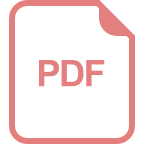
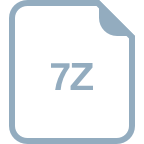
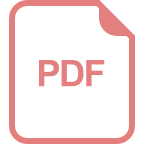
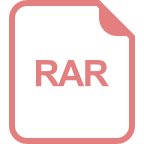
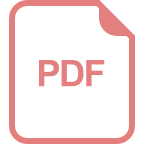
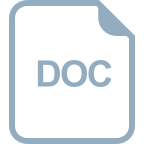
