在程序中引入一个名为readInteger的新方法,方法头为: o私有静态int readInteger() •它不接收任何参数,并且应该返回用户键入的下一个可用整数,邀请它们 重复输入一个整数,直到完成为止。您可以通过复制 从主方法的主体开始,进行少量编辑。注意:该方法不应显示有效的整数 一旦它被接收并且不需要对无效输入进行计数,它应该只返回整数。 •现在使用for循环输入十个语句来替换主方法主体中的语句 整数(在循环体中使用一个调用readInteger的语句),将它们相加 并且在输入处理完成之后显示它们的平均值。
时间: 2023-02-24 11:47:14 浏览: 63
以下是程序代码:
```
private static int readInteger() {
Scanner sc = new Scanner(System.in);
while (!sc.hasNextInt()) {
sc.next();
System.out.println("Invalid input. Please enter an integer.");
}
return sc.nextInt();
}
public static void main(String[] args) {
int sum = 0;
for (int i = 0; i < 10; i++) {
int num = readInteger();
sum += num;
}
System.out.println("The average of the ten numbers is: " + sum / 10);
}
```
这段代码创建了一个名为`readInteger`的方法,它用于读取用户输入的整数。当用户输入无效数据时,它会提示用户重新输入。然后在主方法中,使用一个for循环调用`readInteger`方法十次,并将输入的十个数相加,最后显示它们的平均值。
相关问题
用java写一段词法分析的程序
以下是一个简单的 Java 词法分析器示例,它可以将输入的字符串分解为标记或词法单元:
```java
import java.util.ArrayList;
public class Lexer {
private String input;
private ArrayList<Token> tokens;
private int position;
public Lexer(String input) {
this.input = input;
this.tokens = new ArrayList<Token>();
this.position = 0;
}
public ArrayList<Token> tokenize() throws Exception {
while (position < input.length()) {
char currentChar = input.charAt(position);
if (Character.isDigit(currentChar)) {
tokens.add(new Token(TokenType.INTEGER, readInteger()));
} else if (Character.isLetter(currentChar)) {
tokens.add(new Token(TokenType.IDENTIFIER, readIdentifier()));
} else if (currentChar == '+') {
tokens.add(new Token(TokenType.PLUS, "+"));
position++;
} else if (currentChar == '-') {
tokens.add(new Token(TokenType.MINUS, "-"));
position++;
} else if (currentChar == '*') {
tokens.add(new Token(TokenType.MULTIPLY, "*"));
position++;
} else if (currentChar == '/') {
tokens.add(new Token(TokenType.DIVIDE, "/"));
position++;
} else if (currentChar == '(') {
tokens.add(new Token(TokenType.LPAREN, "("));
position++;
} else if (currentChar == ')') {
tokens.add(new Token(TokenType.RPAREN, ")"));
position++;
} else if (currentChar == '=') {
tokens.add(new Token(TokenType.ASSIGNMENT, "="));
position++;
} else if (currentChar == ';') {
tokens.add(new Token(TokenType.SEMICOLON, ";"));
position++;
} else if (currentChar == ' ') {
position++;
} else {
throw new Exception("Invalid character: " + currentChar);
}
}
return tokens;
}
private String readInteger() {
String result = "";
while (position < input.length() && Character.isDigit(input.charAt(position))) {
result += input.charAt(position);
position++;
}
return result;
}
private String readIdentifier() {
String result = "";
while (position < input.length() && Character.isLetterOrDigit(input.charAt(position))) {
result += input.charAt(position);
position++;
}
return result;
}
}
```
上述代码中,我们定义了 `Lexer` 类,它接受一个字符串作为输入,并将其分解为标记或词法单元。我们使用 `TokenType` 枚举类来表示不同类型的标记,例如整数、标识符、加号、减号等。
在 `tokenize()` 方法中,我们使用一个循环来遍历输入字符串中的每个字符。根据当前字符的类型,我们使用不同的方法来读取标记。例如,如果当前字符是数字,则我们使用 `readInteger()` 方法来读取整数标记。如果当前字符是字母,则我们使用 `readIdentifier()` 方法来读取标识符标记。如果当前字符是运算符或括号,则我们直接将其添加到标记列表中。
在 `readInteger()` 和 `readIdentifier()` 方法中,我们使用另一个循环来读取连续的数字或字母字符,直到遇到非数字或字母字符为止。
最后,我们返回一个标记列表,其中包含输入字符串中的所有标记或词法单元。如果输入字符串中包含无法识别的字符,则我们抛出一个异常。
用java写一个词法分析器
好的,我可以为你提供一个基于 Java 的简单词法分析器实现。你可以参考以下代码:
```java
public class Lexer {
private String input;
private int currentPosition;
public Lexer(String input) {
this.input = input;
this.currentPosition = 0;
}
public Token getNextToken() {
while (currentPosition < input.length()) {
char currentChar = input.charAt(currentPosition);
if (Character.isDigit(currentChar)) {
return new Token(TokenType.INTEGER, readInteger());
}
if (Character.isLetter(currentChar)) {
return new Token(TokenType.IDENTIFIER, readIdentifier());
}
if (currentChar == '+') {
currentPosition++;
return new Token(TokenType.PLUS, "+");
}
if (currentChar == '-') {
currentPosition++;
return new Token(TokenType.MINUS, "-");
}
if (currentChar == '*') {
currentPosition++;
return new Token(TokenType.MULTIPLY, "*");
}
if (currentChar == '/') {
currentPosition++;
return new Token(TokenType.DIVIDE, "/");
}
if (currentChar == '(') {
currentPosition++;
return new Token(TokenType.LEFT_PAREN, "(");
}
if (currentChar == ')') {
currentPosition++;
return new Token(TokenType.RIGHT_PAREN, ")");
}
if (currentChar == '=') {
currentPosition++;
return new Token(TokenType.EQUALS, "=");
}
if (currentChar == ';') {
currentPosition++;
return new Token(TokenType.SEMICOLON, ";");
}
if (Character.isWhitespace(currentChar)) {
skipWhitespace();
continue;
}
throw new RuntimeException("Invalid token: " + currentChar);
}
return new Token(TokenType.EOF, "");
}
private String readInteger() {
StringBuilder sb = new StringBuilder();
while (currentPosition < input.length() && Character.isDigit(input.charAt(currentPosition))) {
sb.append(input.charAt(currentPosition));
currentPosition++;
}
return sb.toString();
}
private String readIdentifier() {
StringBuilder sb = new StringBuilder();
while (currentPosition < input.length() && Character.isLetterOrDigit(input.charAt(currentPosition))) {
sb.append(input.charAt(currentPosition));
currentPosition++;
}
return sb.toString();
}
private void skipWhitespace() {
while (currentPosition < input.length() && Character.isWhitespace(input.charAt(currentPosition))) {
currentPosition++;
}
}
}
```
这是一个简单的词法分析器,它可以识别整数、标识符、运算符等。你可以根据需要修改或扩展它以适应你的具体需求。
相关推荐
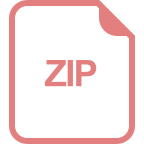
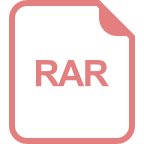
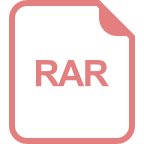




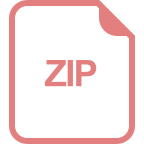
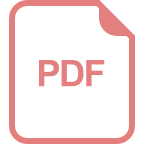
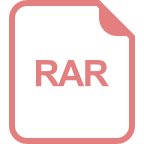
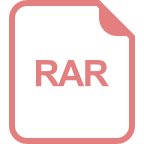
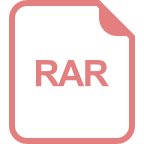
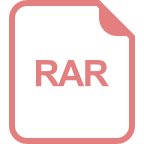
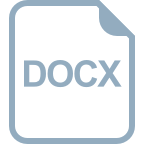