用c语言编程:定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年问题。
时间: 2023-12-03 09:12:42 浏览: 41
好的,以下是用C语言编写的代码:
```c
#include <stdio.h>
struct Date {
int year;
int month;
int day;
};
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
int dayOfYear(struct Date date) {
int days[] = {0,31,59,90,120,151,181,212,243,273,304,334};
int day = days[date.month - 1] + date.day;
if (isLeapYear(date.year) && date.month > 2) {
day++;
}
return day;
}
int main() {
struct Date date = {2021, 8, 12};
int day = dayOfYear(date);
printf("%d年%d月%d日是%d年的第%d天\n", date.year, date.month, date.day, date.year, day);
return 0;
}
```
代码解析如下:
- 首先定义了一个 `Date` 结构体,包括年、月、日三个字段。
- 然后定义了一个 `isLeapYear` 函数,用于判断闰年。如果该年能被4整除但不能被100整除,或者能被400整除,则为闰年。
- 接着定义了一个 `dayOfYear` 函数,用于计算该日在本年中是第几天。首先定义了一个每个月份累计天数的数组 `days`,然后根据输入的日期计算出该日期距离该年1月1日的天数,然后根据是否闰年以及当前月份是否大于2月来判断是否需要加上一个闰年的2月29日的天数。
- 最后在 `main` 函数中定义了一个日期变量 `date`,计算出该日期在本年中的天数,并输出结果。
注意,在实际使用中,可能需要增加一些输入校验和错误处理的代码,以保证程序的健壮性。
相关推荐
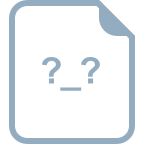
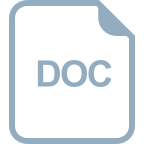
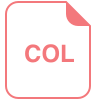
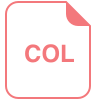
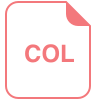
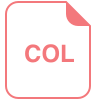
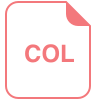









