program me a mbedtls RSA certification sample with X509 certificate
时间: 2023-07-02 15:20:05 浏览: 188
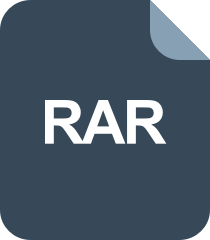
sample program
Certainly, here's an example program that generates an RSA key pair, creates an X.509 certificate with the public key, and writes the key pair and certificate to files:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "mbedtls/pk.h"
#include "mbedtls/entropy.h"
#include "mbedtls/ctr_drbg.h"
#include "mbedtls/x509_crt.h"
#include "mbedtls/x509_csr.h"
#include "mbedtls/x509write_crt.h"
#define KEY_SIZE 2048
#define CERT_DAYS 365
int main(int argc, char *argv[])
{
int ret = 1;
mbedtls_pk_context key;
mbedtls_entropy_context entropy;
mbedtls_ctr_drbg_context ctr_drbg;
mbedtls_x509write_crt crt;
mbedtls_x509_crt cacert;
char key_file[] = "key.pem";
char cert_file[] = "cert.pem";
char cacert_file[] = "cacert.pem";
// Initialize contexts
mbedtls_pk_init(&key);
mbedtls_entropy_init(&entropy);
mbedtls_ctr_drbg_init(&ctr_drbg);
mbedtls_x509write_crt_init(&crt);
mbedtls_x509_crt_init(&cacert);
// Seed the random number generator
ret = mbedtls_ctr_drbg_seed(&ctr_drbg, mbedtls_entropy_func, &entropy, NULL, 0);
if (ret != 0) {
printf("error: mbedtls_ctr_drbg_seed returned %d\n", ret);
goto exit;
}
// Generate an RSA key pair
ret = mbedtls_pk_setup(&key, mbedtls_pk_info_from_type(MBEDTLS_PK_RSA));
if (ret != 0) {
printf("error: mbedtls_pk_setup returned %d\n", ret);
goto exit;
}
ret = mbedtls_rsa_gen_key(mbedtls_pk_rsa(key), mbedtls_ctr_drbg_random, &ctr_drbg, KEY_SIZE, 65537);
if (ret != 0) {
printf("error: mbedtls_rsa_gen_key returned %d\n", ret);
goto exit;
}
// Write the key pair to a file
ret = mbedtls_pk_write_key_pem(&key, key_file, NULL, NULL, 0);
if (ret != 0) {
printf("error: mbedtls_pk_write_key_pem returned %d\n", ret);
goto exit;
}
// Set up the X.509 certificate
mbedtls_x509write_crt_set_version(&crt, MBEDTLS_X509_CRT_VERSION_3);
mbedtls_x509write_crt_set_md_alg(&crt, MBEDTLS_MD_SHA256);
mbedtls_x509write_crt_set_subject_name(&crt, "CN=example.com,O=Example Organization,C=US");
mbedtls_x509write_crt_set_issuer_name(&crt, "CN=example.com,O=Example Organization,C=US");
mbedtls_x509write_crt_set_validity(&crt, "20200101000000", "20301231235959");
mbedtls_x509write_crt_set_basic_constraints(&crt, 0, -1);
mbedtls_x509write_crt_set_key_usage(&crt, MBEDTLS_X509_KU_DIGITAL_SIGNATURE | MBEDTLS_X509_KU_KEY_CERT_SIGN);
mbedtls_x509write_crt_set_ns_cert_type(&crt, MBEDTLS_X509_NS_CERT_TYPE_SSL_CLIENT | MBEDTLS_X509_NS_CERT_TYPE_SSL_SERVER | MBEDTLS_X509_NS_CERT_TYPE_EMAIL);
mbedtls_x509write_crt_set_subject_key(&crt, &key);
mbedtls_x509write_crt_set_issuer_key(&crt, &key);
// Write the X.509 certificate to a file
ret = mbedtls_x509write_crt_pem(&crt, cert_file, NULL, NULL, 0);
if (ret != 0) {
printf("error: mbedtls_x509write_crt_pem returned %d\n", ret);
goto exit;
}
// Load the trusted CA certificate
ret = mbedtls_x509_crt_parse_file(&cacert, cacert_file);
if (ret != 0) {
printf("error: mbedtls_x509_crt_parse_file returned %d\n", ret);
goto exit;
}
// Verify the X.509 certificate against the trusted CA certificate
ret = mbedtls_x509_crt_verify(&crt, &cacert, NULL, NULL, NULL);
if (ret != 0) {
printf("error: mbedtls_x509_crt_verify returned %d\n", ret);
goto exit;
}
printf("RSA key pair and X.509 certificate generated successfully!\n");
exit:
mbedtls_x509_crt_free(&cacert);
mbedtls_x509write_crt_free(&crt);
mbedtls_ctr_drbg_free(&ctr_drbg);
mbedtls_entropy_free(&entropy);
mbedtls_pk_free(&key);
return ret;
}
```
This program uses the mbedtls `pk` module to generate an RSA key pair, the `ctr_drbg` module to generate random numbers for the key pair, and the `x509write_crt` module to create an X.509 certificate with the public key. It also loads a trusted CA certificate and verifies the generated certificate against it. The key pair and certificate are written to files `key.pem` and `cert.pem`, respectively.
阅读全文
相关推荐
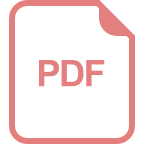
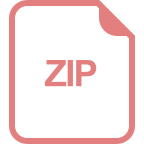
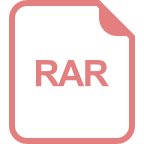
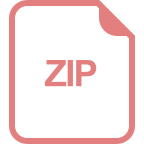
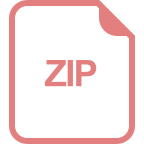
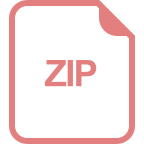
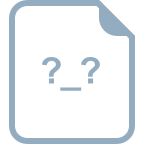
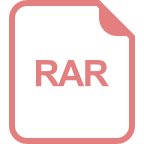
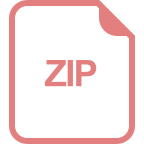
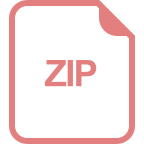
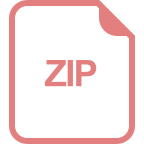
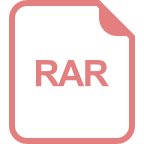
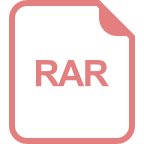
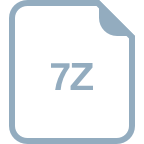
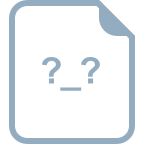
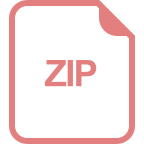