vue3 路由跳转并传参
时间: 2023-09-09 15:12:59 浏览: 42
在Vue 3中,你可以使用Vue Router来进行路由跳转并传递参数。下面是一个简单的示例:
首先,确保你已经安装并配置了Vue Router。
1. 在你的路由文件中(通常是`router.js`),定义路由和对应的组件:
```javascript
import { createRouter, createWebHistory } from 'vue-router';
import Home from './views/Home.vue';
import Profile from './views/Profile.vue';
const routes = [
{
path: '/',
name: 'home',
component: Home
},
{
path: '/profile/:id',
name: 'profile',
component: Profile
}
];
const router = createRouter({
history: createWebHistory(),
routes
});
export default router;
```
2. 在你的组件中,使用`router-link`标签进行路由跳转,并传递参数:
```html
<template>
<div>
<router-link :to="{ name: 'profile', params: { id: userId }}">Go to Profile</router-link>
</div>
</template>
<script>
export default {
data() {
return {
userId: 123 // 假设这个是动态获取的用户ID
}
}
}
</script>
```
3. 在接收参数的组件中,使用`$route.params`来获取传递的参数:
```html
<template>
<div>
<h2>Profile Page</h2>
<p>User ID: {{ $route.params.id }}</p>
</div>
</template>
<script>
export default {
mounted() {
console.log(this.$route.params.id); // 输出传递的参数
}
}
</script>
```
通过上述步骤,你就可以在Vue 3中实现路由跳转并传递参数了。在示例中,我们使用了`router-link`来进行跳转,并在`params`对象中传递了参数。在目标组件中,使用`$route.params`来获取参数。
相关推荐
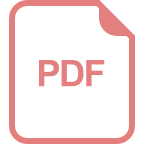
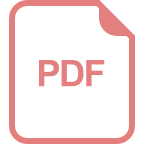














