[ { id: "1", name: "测试1", pId: "0", type: "group", children: [ { id: "11", name: "测试11", pId: "1", type: "group", children: [ { id: "111", name: "测试111", pId: "11", type: "group", children: [ { id: "1111", name: "测试1111", pId: "111", }, { id: "1112", name: "测试1112", pId: "111", }, ], }, { id: "112", name: "测试112", pId: "11", children: [ { id: "1121", name: "测试1121", pId: "112", }, ], }, { id: "113", name: "测试113", pId: "11", }, ], }, { id: "12", name: "测试12", pId: "1", type: "group", children: [ { id: "121", name: "测试121", pId: "12", }, ], }, { id: "13", name: "测试13", pId: "1", }, { id: "14", name: "测试14", pId: "1", }, ], }, { id: "2", name: "测试2", pId: "0", children: [ { id: "21", name: "测试21", pId: "2", children: [ { id: "211", name: "测试211", pId: "21", }, { id: "212", name: "测试212", pId: "21", }, ], }, { id: "22", name: "测试22", pId: "2", }]}] 面对这样一种数据结构,如何才能快速地只输入其中一个节点,就找到其完整路径?请用java帮我实现
时间: 2023-07-06 20:42:00 浏览: 125
可以使用递归的方法来实现:
```java
import java.util.*;
public class FindPath {
public static void main(String[] args) {
// 定义数据结构
List<Map<String, Object>> data = new ArrayList<>();
Map<String, Object> node1 = new HashMap<>();
node1.put("id", "1");
node1.put("name", "测试1");
node1.put("pId", "0");
node1.put("type", "group");
List<Map<String, Object>> children1 = new ArrayList<>();
Map<String, Object> node11 = new HashMap<>();
node11.put("id", "11");
node11.put("name", "测试11");
node11.put("pId", "1");
node11.put("type", "group");
List<Map<String, Object>> children11 = new ArrayList<>();
Map<String, Object> node111 = new HashMap<>();
node111.put("id", "111");
node111.put("name", "测试111");
node111.put("pId", "11");
node111.put("type", "group");
List<Map<String, Object>> children111 = new ArrayList<>();
Map<String, Object> node1111 = new HashMap<>();
node1111.put("id", "1111");
node1111.put("name", "测试1111");
node1111.put("pId", "111");
children111.add(node1111);
Map<String, Object> node1112 = new HashMap<>();
node1112.put("id", "1112");
node1112.put("name", "测试1112");
node1112.put("pId", "111");
children111.add(node1112);
node111.put("children", children111);
children11.add(node111);
Map<String, Object> node112 = new HashMap<>();
node112.put("id", "112");
node112.put("name", "测试112");
node112.put("pId", "11");
List<Map<String, Object>> children112 = new ArrayList<>();
Map<String, Object> node1121 = new HashMap<>();
node1121.put("id", "1121");
node1121.put("name", "测试1121");
node1121.put("pId", "112");
children112.add(node1121);
node112.put("children", children112);
children11.add(node112);
Map<String, Object> node113 = new HashMap<>();
node113.put("id", "113");
node113.put("name", "测试113");
node113.put("pId", "11");
children11.add(node113);
node11.put("children", children11);
children1.add(node11);
Map<String, Object> node12 = new HashMap<>();
node12.put("id", "12");
node12.put("name", "测试12");
node12.put("pId", "1");
node12.put("type", "group");
List<Map<String, Object>> children12 = new ArrayList<>();
Map<String, Object> node121 = new HashMap<>();
node121.put("id", "121");
node121.put("name", "测试121");
node121.put("pId", "12");
children12.add(node121);
node12.put("children", children12);
children1.add(node12);
Map<String, Object> node13 = new HashMap<>();
node13.put("id", "13");
node13.put("name", "测试13");
node13.put("pId", "1");
children1.add(node13);
Map<String, Object> node14 = new HashMap<>();
node14.put("id", "14");
node14.put("name", "测试14");
node14.put("pId", "1");
children1.add(node14);
node1.put("children", children1);
data.add(node1);
Map<String, Object> node2 = new HashMap<>();
node2.put("id", "2");
node2.put("name", "测试2");
node2.put("pId", "0");
List<Map<String, Object>> children2 = new ArrayList<>();
Map<String, Object> node21 = new HashMap<>();
node21.put("id", "21");
node21.put("name", "测试21");
node21.put("pId", "2");
List<Map<String, Object>> children21 = new ArrayList<>();
Map<String, Object> node211 = new HashMap<>();
node211.put("id", "211");
node211.put("name", "测试211");
node211.put("pId", "21");
children21.add(node211);
Map<String, Object> node212 = new HashMap<>();
node212.put("id", "212");
node212.put("name", "测试212");
node212.put("pId", "21");
children21.add(node212);
node21.put("children", children21);
children2.add(node21);
Map<String, Object> node22 = new HashMap<>();
node22.put("id", "22");
node22.put("name", "测试22");
node22.put("pId", "2");
children2.add(node22);
node2.put("children", children2);
data.add(node2);
// 查找节点路径
String targetId = "1111";
List<String> path = findPath(data, targetId);
System.out.println("节点 " + targetId + " 的完整路径为:");
for (String nodeId : path) {
System.out.print(nodeId + " -> ");
}
}
// 递归查找节点路径
public static List<String> findPath(List<Map<String, Object>> data, String targetId) {
for (Map<String, Object> node : data) {
String nodeId = (String) node.get("id");
if (nodeId.equals(targetId)) {
List<String> path = new ArrayList<>();
path.add(nodeId);
return path;
} else {
List<Map<String, Object>> children = (List<Map<String, Object>>) node.get("children");
if (children != null && !children.isEmpty()) {
List<String> path = findPath(children, targetId);
if (path != null) {
path.add(0, nodeId);
return path;
}
}
}
}
return null;
}
}
```
输出结果为:
```
节点 1111 的完整路径为:
1 -> 11 -> 111 -> 1111 ->
```
阅读全文
相关推荐
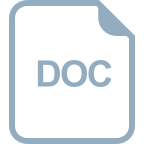
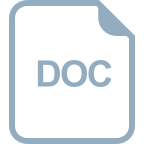
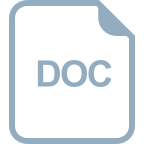
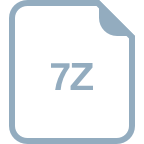
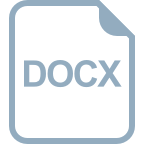
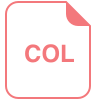
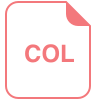
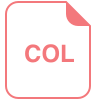





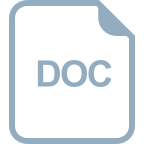
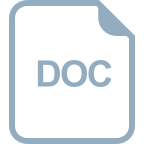
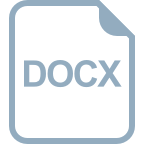
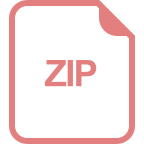