C Sharp 线程锁示例
时间: 2023-10-22 21:08:08 浏览: 36
Sure, I can provide you with a C# threading example that uses locks. Here is an example code snippet:
```csharp
using System;
using System.Threading;
class Program
{
private static readonly object _lock = new object();
static void Main(string[] args)
{
Thread thread1 = new Thread(() =>
{
lock (_lock)
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine($"Thread 1 - count: {i}");
Thread.Sleep(200);
}
}
});
Thread thread2 = new Thread(() =>
{
lock (_lock)
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine($"Thread 2 - count: {i}");
Thread.Sleep(200);
}
}
});
thread1.Start();
thread2.Start();
thread1.Join();
thread2.Join();
Console.ReadKey();
}
}
```
In this example, we have two threads running and both of them use a lock object to ensure that they execute the block of code inside the lock statement atomically. The lock object is created using the `object` type and it simply serves as a mutual exclusion mechanism to prevent concurrent access to the shared resource (in this case, the console output).
Hope that helps! Let me know if you need anything else.
相关推荐
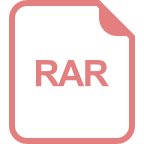
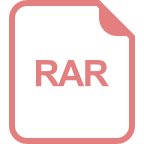
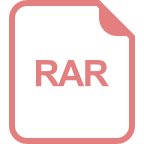











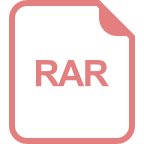
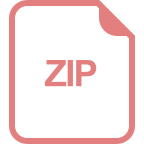
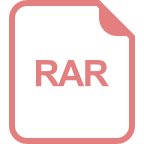
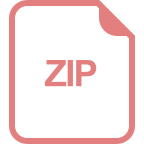