用mfc做一个翻图游戏
时间: 2024-01-30 12:03:06 浏览: 120
好的,下面是一个简单的 MFC 翻图游戏的实现:
1. 新建一个 MFC 应用程序,选择对话框作为应用程序类型。
2. 在对话框中添加一个 Picture Control,用于显示游戏界面。
3. 在对话框中添加一个按钮,用于开始游戏。
4. 在对话框类的头文件中添加以下成员变量:
```
private:
CBitmap m_bitmap[16]; // 存储图片
int m_order[16]; // 存储图片的顺序
int m_emptyIndex; // 空白格子的索引
```
5. 在 OnInitDialog() 函数中加载图片并打乱顺序:
```
// 加载图片
for (int i = 0; i < 16; i++)
{
CString str;
str.Format(_T("IDB_BITMAP%d"), i + 1);
m_bitmap[i].LoadBitmap(str);
}
// 打乱顺序
srand((unsigned)time(NULL));
for (int i = 0; i < 16; i++)
{
m_order[i] = i;
}
for (int i = 15; i > 0; i--)
{
int j = rand() % (i + 1);
int tmp = m_order[i];
m_order[i] = m_order[j];
m_order[j] = tmp;
}
m_emptyIndex = 15;
```
6. 在 OnPaint() 函数中绘制图片:
```
CPaintDC dc(this);
CRect rect;
GetDlgItem(IDC_PICTURE)->GetClientRect(rect);
int width = rect.Width() / 4;
int height = rect.Height() / 4;
for (int i = 0; i < 16; i++)
{
int row = i / 4;
int col = i % 4;
int x = col * width;
int y = row * height;
if (i == m_emptyIndex)
{
dc.FillSolidRect(x, y, width, height, RGB(255, 255, 255));
}
else
{
CDC memDC;
memDC.CreateCompatibleDC(&dc);
memDC.SelectObject(&m_bitmap[m_order[i]]);
dc.BitBlt(x, y, width, height, &memDC, 0, 0, SRCCOPY);
}
}
```
7. 在 OnLButtonDown() 函数中处理点击事件:
```
CPoint point = pWnd->GetMessagePos();
ScreenToClient(&point);
int row = point.y / height;
int col = point.x / width;
int index = row * 4 + col;
if (col > 0 && index - 1 == m_emptyIndex) // 左
{
int tmp = m_order[index];
m_order[index] = m_order[m_emptyIndex];
m_order[m_emptyIndex] = tmp;
m_emptyIndex = index;
Invalidate();
}
else if (col < 3 && index + 1 == m_emptyIndex) // 右
{
int tmp = m_order[index];
m_order[index] = m_order[m_emptyIndex];
m_order[m_emptyIndex] = tmp;
m_emptyIndex = index;
Invalidate();
}
else if (row > 0 && index - 4 == m_emptyIndex) // 上
{
int tmp = m_order[index];
m_order[index] = m_order[m_emptyIndex];
m_order[m_emptyIndex] = tmp;
m_emptyIndex = index;
Invalidate();
}
else if (row < 3 && index + 4 == m_emptyIndex) // 下
{
int tmp = m_order[index];
m_order[index] = m_order[m_emptyIndex];
m_order[m_emptyIndex] = tmp;
m_emptyIndex = index;
Invalidate();
}
```
8. 在 OnBnClickedStart() 函数中重新打乱顺序并重绘:
```
// 打乱顺序
srand((unsigned)time(NULL));
for (int i = 0; i < 16; i++)
{
m_order[i] = i;
}
for (int i = 15; i > 0; i--)
{
int j = rand() % (i + 1);
int tmp = m_order[i];
m_order[i] = m_order[j];
m_order[j] = tmp;
}
m_emptyIndex = 15;
// 重绘
Invalidate();
```
这样就实现了一个简单的翻图游戏。
阅读全文
相关推荐




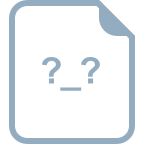













