帮我用java 写一个生成树形结构的json代码
时间: 2023-08-31 12:25:14 浏览: 76
### 回答1:
生成树形结构的 JSON 代码可以使用递归方法来实现。
以下是一个示例代码:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
public class TreeNode {
String name;
List<TreeNode> children;
public TreeNode(String name) {
this.name = name;
this.children = new ArrayList<>();
}
public void addChild(TreeNode child) {
this.children.add(child);
}
}
public class TreeGenerator {
public static void main(String[] args) {
// 创建根节点
TreeNode root = new TreeNode("root");
// 创建子节点
TreeNode node1 = new TreeNode("node1");
TreeNode node2 = new TreeNode("node2");
TreeNode node3 = new TreeNode("node3");
// 将子节点添加到根节点的 children 中
root.addChild(node1);
root.addChild(node2);
root.addChild(node3);
// 创建孙子节点
TreeNode node4 = new TreeNode("node4");
TreeNode node5 = new TreeNode("node5");
TreeNode node6 = new TreeNode("node6");
// 将孙子节点添加到第一个子节点的 children 中
node1.addChild(node4);
node1.addChild(node5);
node1.addChild(node6);
// 使用 Jackson 库将树形结构转换为 JSON 字符串
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(root);
System.out.println(json);
}
}
```
在这个代码中,我们定义了一个 TreeNode 类表示树节点,其中包含了节点名称和子节点的列表。然后我们使用递归的方法来构建树形结构,并使用 Jackson 库将其转换为 JSON 字符串。
运行这个代码后,你将得到一个类似于下面的输出:
```json
### 回答2:
根据您的要求,我为您提供一个使用Java编写的生成树形结构的JSON代码示例。
首先,我们需要定义一个节点类Node,该类包含节点的标识符id、节点名称name以及子节点的列表children。
```java
import java.util.ArrayList;
import java.util.List;
class Node {
private String id;
private String name;
private List<Node> children;
public Node(String id, String name) {
this.id = id;
this.name = name;
this.children = new ArrayList<>();
}
public void addChild(Node node) {
children.add(node);
}
// 这里省略了getter和setter方法
// 将节点及其子节点转化为JSON格式的字符串
public String toJson() {
StringBuilder json = new StringBuilder();
json.append("{");
json.append("\"id\":\"").append(id).append("\",");
json.append("\"name\":\"").append(name).append("\"");
if (!children.isEmpty()) {
json.append(",\"children\":[");
for (int i = 0; i < children.size(); i++) {
if (i > 0) {
json.append(",");
}
json.append(children.get(i).toJson());
}
json.append("]");
}
json.append("}");
return json.toString();
}
}
```
接下来,我们可以使用Node类构建树形结构的节点,并将其转化为JSON格式的字符串输出。
```java
public class Main {
public static void main(String[] args) {
Node root = new Node("1", "Root");
Node child1 = new Node("2", "Child 1");
Node child2 = new Node("3", "Child 2");
Node child3 = new Node("4", "Child 3");
Node grandchild1 = new Node("5", "Grandchild 1");
Node grandchild2 = new Node("6", "Grandchild 2");
child1.addChild(grandchild1);
child2.addChild(grandchild2);
root.addChild(child1);
root.addChild(child2);
root.addChild(child3);
String json = root.toJson();
System.out.println(json);
}
}
```
以上代码通过创建节点对象,添加子节点,并使用toJson方法将节点转化为JSON格式的字符串。您可以根据自己的需求修改节点的属性和结构。
### 回答3:
Sure, 下面是一个使用Java编写的生成树形结构JSON的示例代码:
```java
import com.google.gson.JsonArray;
import com.google.gson.JsonObject;
public class TreeNode {
private int id;
private String name;
private TreeNode parent;
private List<TreeNode> children;
public TreeNode(int id, String name) {
this.id = id;
this.name = name;
this.children = new ArrayList<>();
}
public void setParent(TreeNode parent) {
this.parent = parent;
}
public void addChild(TreeNode child) {
child.setParent(this);
children.add(child);
}
public JsonObject toJson() {
JsonObject json = new JsonObject();
json.addProperty("id", id);
json.addProperty("name", name);
if (parent != null) {
json.addProperty("parentId", parent.getId());
}
if (children.size() > 0) {
JsonArray childrenArray = new JsonArray();
for (TreeNode child : children) {
childrenArray.add(child.toJson());
}
json.add("children", childrenArray);
}
return json;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public TreeNode getParent() {
return parent;
}
public List<TreeNode> getChildren() {
return children;
}
public static void main(String[] args) {
TreeNode root = new TreeNode(1, "Root");
TreeNode node1 = new TreeNode(2, "Node 1");
TreeNode node2 = new TreeNode(3, "Node 2");
TreeNode node3 = new TreeNode(4, "Node 3");
root.addChild(node1);
root.addChild(node2);
node1.addChild(node3);
JsonObject json = root.toJson();
System.out.println(json.toString());
}
}
```
在这个示例代码中,TreeNode类表示树中的一个节点,包含id、name、parent和children等属性。节点可以通过addChild()方法添加子节点,并通过toJson()方法将节点转换为JSON对象。使用Gson库可以将JSON对象打印出来。
在main方法中,我们创建了一个树形结构,并将根节点转换为JSON对象并打印出来。您可以根据需要修改节点的属性和结构。希望这个代码对您有帮助!
相关推荐
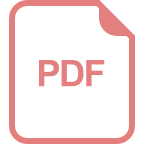
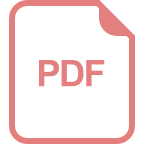














