设计一个用于人事管理的People(人员)类。考虑到通用性,只抽象出所有类型人员都具有的属性:number(编号)、sex(性别)、birthday(出生日期)、id(身份证号)、ntotalnumber(人员总数)。其中“人员总数”用静态成员变量表示,并用一个静态成员函数输出总人数;“出生日期”声明为一个“日期类(calendar)”成员对象,作为People(人员)类的封闭类对象。用成员函数实现对人信息的录入和显示。要求包括:构造函数和析构函数、复制构造函数、内敛成员函数、静态成员函数。
时间: 2024-01-17 11:05:16 浏览: 127
好的,根据您的要求,我来设计一个用于人事管理的People(人员)类。
```cpp
#include <iostream>
using namespace std;
class People {
private:
int number; // 编号
string sex; // 性别
string id; // 身份证号
static int ntotalnumber; // 人员总数
class calendar { // 出生日期,作为People(人员)类的封闭类对象
public:
int year;
int month;
int day;
calendar(int y, int m, int d) : year(y), month(m), day(d) {}
void display() {
cout << year << "-" << month << "-" << day << endl;
}
} birthday;
public:
People(int n, string s, string i, int y, int m, int d) : number(n), sex(s), id(i), birthday(y, m, d) {
ntotalnumber++;
}
People(const People& p) : number(p.number), sex(p.sex), id(p.id), birthday(p.birthday.year, p.birthday.month, p.birthday.day) {}
~People() {
ntotalnumber--;
}
void display() {
cout << "编号:" << number << endl;
cout << "性别:" << sex << endl;
cout << "身份证号:" << id << endl;
cout << "出生日期:";
birthday.display();
}
static void displayTotalNumber() {
cout << "总人数:" << ntotalnumber << endl;
}
};
int People::ntotalnumber = 0; // 初始化静态成员变量
int main() {
People p1(1, "男", "123456789012345678", 1990, 10, 1);
People p2(2, "女", "123456789012345679", 1995, 3, 15);
People::displayTotalNumber(); // 输出总人数
p1.display(); // 输出p1的信息
p2.display(); // 输出p2的信息
return 0;
}
```
以上是一个比较简单的People(人员)类的设计,包括了构造函数和析构函数、复制构造函数、内联成员函数、静态成员函数等。其中,出生日期使用了封闭类对象的方式,静态成员变量和静态成员函数用于统计和输出总人数。
阅读全文
相关推荐
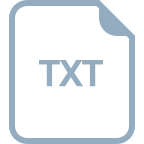


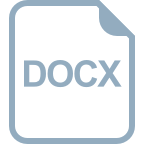
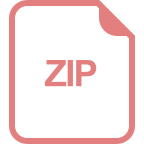
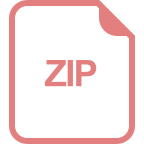
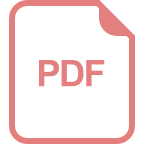