用cpp语言制作一个程序,要求写出完整的代码,该程序先读取一个单词,该单词以字母开头,包括字母数字的任意序列.然后程序检查该单词是否位于树中.如果是,就使用半张开技术,重新组织树,并给这一单词的出现次数加1.如果没有在树中找到单词,就为该单词创建一个新的叶子节点,将其插入树中.处理完所有的单词后,对树进行中序遍历,计算所有节点的频率,再把这些频率计数加在一起并输出,作为树中单词个数和文件中单词个数的最后结果.
时间: 2023-02-05 07:59:31 浏览: 52
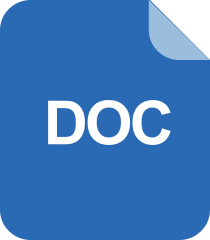
一个最简单的MFC程序实例.doc

这里是一个用 C++ 语言实现的代码示例,它演示了如何使用树来存储单词,并实现以上要求:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义树节点结构体
struct TreeNode {
string word; // 单词
int count; // 单词出现次数
TreeNode* left; // 左子树
TreeNode* right; // 右子树
TreeNode(string w) : word(w), count(1), left(nullptr), right(nullptr) {}
};
// 插入单词到树中
void insert(TreeNode*& root, string word) {
if (root == nullptr) {
root = new TreeNode(word);
} else if (word < root->word) {
insert(root->left, word);
} else if (word > root->word) {
insert(root->right, word);
} else {
root->count++;
}
}
// 中序遍历树并计算总频率
int traverse(TreeNode* root) {
if (root == nullptr) {
return 0;
}
int count = traverse(root->left) + root->count;
cout << root->word << ": " << root->count << endl;
count += traverse(root->right);
return count;
}
int main() {
TreeNode* root = nullptr;
// 读取输入并插入树中
string word;
while (cin >> word) {
insert(root, word);
}
// 计算总频率并输出结果
int total = traverse(root);
cout << "Total: " << total << endl;
return 0;
}
```
希望这个示例代码能帮到你!
阅读全文
相关推荐
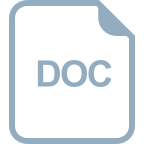
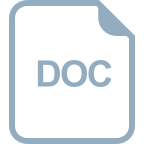
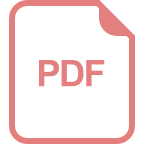
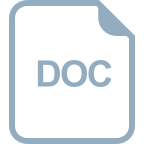
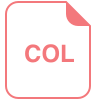
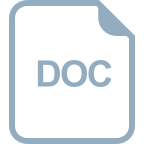
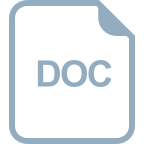
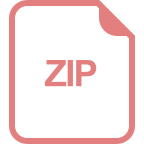
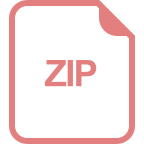
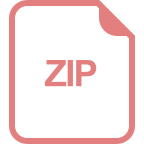
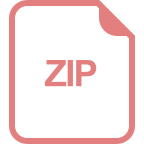
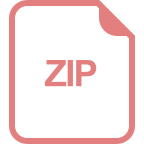
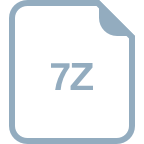
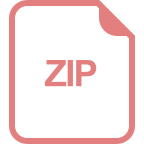
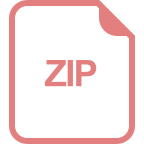
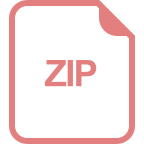
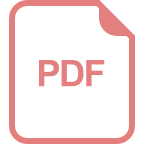