嵌入式linux下LCD编程显示200*120bmp图片让它在800*480的显示屏上动态显示
时间: 2023-06-15 17:03:22 浏览: 78
在嵌入式Linux下,可以使用FrameBuffer来控制LCD屏幕的显示。以下是一个简单的步骤:
1. 确认LCD屏幕的型号和分辨率,并且在系统中加载相应的驱动程序。
2. 将200*120的BMP图片转换成RGB格式,并将其存储在内存中。
3. 使用FrameBuffer的API,将RGB数据写入FrameBuffer的显存中。
4. 使用定时器或线程来不断更新显存中的数据,从而实现动态显示效果。
下面是一个简单的示例代码,仅供参考:
```c
#include <fcntl.h>
#include <linux/fb.h>
#include <stdio.h>
#include <sys/ioctl.h>
#include <sys/mman.h>
int main(int argc, char* argv[])
{
int fb_fd;
struct fb_var_screeninfo vinfo;
struct fb_fix_screeninfo finfo;
char* fbp = 0;
int x, y;
long int location = 0;
fb_fd = open("/dev/fb0", O_RDWR);
if (fb_fd == -1) {
printf("Error: cannot open framebuffer device.\n");
return 1;
}
if (ioctl(fb_fd, FBIOGET_FSCREENINFO, &finfo) == -1) {
printf("Error: cannot get fixed screen info.\n");
return 1;
}
if (ioctl(fb_fd, FBIOGET_VSCREENINFO, &vinfo) == -1) {
printf("Error: cannot get variable screen info.\n");
return 1;
}
int width = vinfo.xres;
int height = vinfo.yres;
long int screensize = finfo.smem_len;
fbp = (char*)mmap(0, screensize, PROT_READ | PROT_WRITE, MAP_SHARED, fb_fd, 0);
// load 200x120 BMP image
char* image_data = load_bmp("image.bmp", 200, 120);
// convert BMP image to RGB format
char* rgb_data = convert_to_rgb(image_data, 200, 120);
// copy RGB data to FrameBuffer
for (y = 0; y < height; y++) {
for (x = 0; x < width; x++) {
location = (x + vinfo.xoffset) * (vinfo.bits_per_pixel / 8) +
(y + vinfo.yoffset) * finfo.line_length;
*(fbp + location) = rgb_data[(x + y * width) * 3];
*(fbp + location + 1) = rgb_data[(x + y * width) * 3 + 1];
*(fbp + location + 2) = rgb_data[(x + y * width) * 3 + 2];
*(fbp + location + 3) = 0;
}
}
// update FrameBuffer at regular intervals to create animation
while (1) {
// update RGB data
update_rgb_data(rgb_data, 200, 120);
// copy RGB data to FrameBuffer
for (y = 0; y < height; y++) {
for (x = 0; x < width; x++) {
location = (x + vinfo.xoffset) * (vinfo.bits_per_pixel / 8) +
(y + vinfo.yoffset) * finfo.line_length;
*(fbp + location) = rgb_data[(x + y * width) * 3];
*(fbp + location + 1) = rgb_data[(x + y * width) * 3 + 1];
*(fbp + location + 2) = rgb_data[(x + y * width) * 3 + 2];
*(fbp + location + 3) = 0;
}
}
usleep(10000); // sleep for 10ms
}
munmap(fbp, screensize);
close(fb_fd);
return 0;
}
```
需要注意的是,这只是一个简单的示例代码,实际情况可能会更加复杂。例如,需要考虑图片的缩放和旋转,以及多线程和多进程的并发控制等。
相关推荐
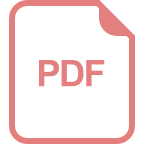
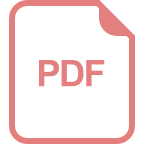














