c语言走迷宫程序怎么编
时间: 2024-03-02 10:49:06 浏览: 82
编写 C 语言走迷宫程序需要以下步骤:
1. 定义迷宫地图:可以使用二维数组来表示迷宫地图,其中 0 表示可以通行的路,1 表示墙壁,2 表示起点,3 表示终点。
2. 初始化起点和终点:可以在地图中找到起点和终点的位置,并将其标记为 2 和 3。
3. 定义一个栈:用于保存路径信息。
4. 编写搜索算法:可以使用深度优先搜索(DFS)或广度优先搜索(BFS)算法在地图中寻找通往终点的路径,将路径信息保存到栈中。
5. 输出结果:将栈中保存的路径信息输出,即可找到从起点到终点的最短路径。
下面是一个简单的 C 语言迷宫求解程序的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROW 5 // 迷宫行数
#define COL 5 // 迷宫列数
int maze[ROW][COL] = {
{1, 1, 1, 1, 1},
{0, 0, 0, 0, 1},
{1, 1, 1, 0, 1},
{1, 0, 0, 0, 1},
{1, 1, 1, 1, 1}
}; // 迷宫地图
typedef struct {
int row; // 行坐标
int col; // 列坐标
} Position;
typedef struct Stack {
Position pos; // 当前位置
struct Stack *next; // 下一个元素
} Stack;
void push(Stack **top, Position pos) { // 入栈操作
Stack *new_node = (Stack *)malloc(sizeof(Stack));
new_node->pos = pos;
new_node->next = *top;
*top = new_node;
}
void pop(Stack **top) { // 出栈操作
Stack *temp = *top;
*top = (*top)->next;
free(temp);
}
Position top(Stack *top) { // 获取栈顶元素
return top->pos;
}
int is_empty(Stack *top) { // 判断栈是否为空
return top == NULL;
}
int is_valid(int row, int col) { // 判断坐标是否有效
return row >= 0 && row < ROW && col >= 0 && col < COL && maze[row][col] == 0;
}
void print_path(Stack *top) { // 输出路径
while (!is_empty(top)) {
printf("(%d, %d)\n", top->pos.row, top->pos.col);
pop(&top);
}
}
void solve_maze(Position start, Position end) { // 迷宫求解函数
Stack *path = NULL; // 路径栈
push(&path, start); // 将起点入栈
while (!is_empty(path)) {
Position cur = top(path);
if (cur.row == end.row && cur.col == end.col) { // 到达终点,输出路径
print_path(path);
return;
}
// 按照右、下、左、上的顺序检查相邻位置
if (is_valid(cur.row, cur.col + 1)) { // 右
push(&path, (Position){cur.row, cur.col + 1});
maze[cur.row][cur.col + 1] = 1; // 将当前位置标记为已经走过
} else if (is_valid(cur.row + 1, cur.col)) { // 下
push(&path, (Position){cur.row + 1, cur.col});
maze[cur.row + 1][cur.col] = 1;
} else if (is_valid(cur.row, cur.col - 1)) { // 左
push(&path, (Position){cur.row, cur.col - 1});
maze[cur.row][cur.col - 1] = 1;
} else if (is_valid(cur.row - 1, cur.col)) { // 上
push(&path, (Position){cur.row - 1, cur.col});
maze[cur.row - 1][cur.col] = 1;
} else { // 无法继续前进,回溯
pop(&path);
}
}
printf("No path found.\n"); // 没有找到通往终点的路径
}
int main() {
Position start = {1, 0}; // 起点
Position end = {3, 4}; // 终点
solve_maze(start, end);
return 0;
}
```
阅读全文
相关推荐
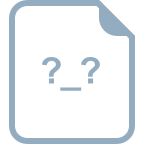
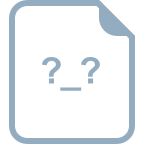
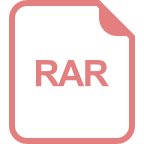
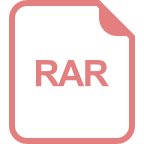
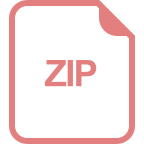
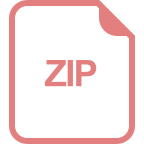
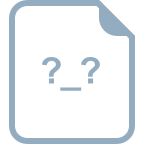
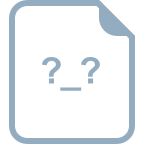