用数据结构写出一个商品销售管理系统的c++源代码
时间: 2023-08-04 12:39:26 浏览: 89
好的,下面是一个简单的商品销售管理系统的 C++ 源代码,其中使用了链表数据结构:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 商品结构体
struct Product {
string name; // 商品名称
double price; // 商品价格
int quantity; // 商品数量
// 构造函数
Product(string n, double p, int q) {
name = n;
price = p;
quantity = q;
}
};
// 节点结构体
struct Node {
Product* product; // 商品指针
Node* next; // 下一个节点指针
// 构造函数
Node(Product* p) {
product = p;
next = nullptr;
}
};
// 链表类
class ProductList {
public:
ProductList() {
head = nullptr;
}
// 添加商品
void addProduct(Product* p) {
Node* newNode = new Node(p);
if (head == nullptr) {
head = newNode;
} else {
Node* cur = head;
while (cur->next != nullptr) {
cur = cur->next;
}
cur->next = newNode;
}
}
// 显示所有商品
void displayProducts() {
Node* cur = head;
while (cur != nullptr) {
cout << cur->product->name << " - $" << cur->product->price << " - " << cur->product->quantity << " in stock" << endl;
cur = cur->next;
}
}
// 查找商品
Product* findProduct(string name) {
Node* cur = head;
while (cur != nullptr) {
if (cur->product->name == name) {
return cur->product;
}
cur = cur->next;
}
return nullptr;
}
private:
Node* head; // 头节点指针
};
int main() {
// 初始化商品列表
ProductList productList;
productList.addProduct(new Product("iPhone", 999.99, 10));
productList.addProduct(new Product("iPad", 799.99, 8));
productList.addProduct(new Product("MacBook", 1299.99, 5));
// 显示所有商品
cout << "All products:" << endl;
productList.displayProducts();
// 查找商品
string productName;
cout << "Enter product name to search: ";
cin >> productName;
Product* product = productList.findProduct(productName);
if (product != nullptr) {
cout << "Product found: " << product->name << " - $" << product->price << " - " << product->quantity << " in stock" << endl;
} else {
cout << "Product not found." << endl;
}
return 0;
}
```
上面的代码中,`Product` 结构体表示商品,`Node` 结构体表示节点,`ProductList` 类表示链表,提供了添加商品、显示所有商品和查找商品的功能。可以根据实际需求进行修改和扩展。
阅读全文
相关推荐












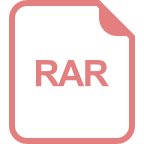


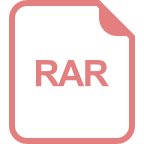

