springboot中实现AioQuickClient
时间: 2024-06-08 22:10:55 浏览: 6
在Spring Boot中实现AioQuickClient,可以使用Netty框架提供的异步非阻塞I/O操作。下面是一个简单的示例代码,你可以根据自己的需求进行修改:
1. 首先,在pom.xml中添加Netty依赖:
```xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.63.Final</version>
</dependency>
```
2. 创建一个AioQuickClient类,用于连接服务器、发送和接收数据:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.ByteToMessageDecoder;
import io.netty.handler.codec.MessageToByteEncoder;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
import java.nio.charset.Charset;
import java.util.List;
public class AioQuickClient {
private String host;
private int port;
private Bootstrap bootstrap;
private EventLoopGroup workerGroup;
private ChannelFuture channelFuture;
public AioQuickClient(String host, int port) {
this.host = host;
this.port = port;
init();
}
private void init() {
bootstrap = new Bootstrap();
workerGroup = new NioEventLoopGroup();
bootstrap.group(workerGroup)
.channel(NioSocketChannel.class)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new StringEncoder(Charset.forName("UTF-8")));
ch.pipeline().addLast(new StringDecoder(Charset.forName("UTF-8")));
// 添加自定义编码器
ch.pipeline().addLast(new MyEncoder());
// 添加自定义解码器
ch.pipeline().addLast(new MyDecoder());
// 添加自定义处理器
ch.pipeline().addLast(new MyHandler());
}
});
}
public void connect() throws InterruptedException {
channelFuture = bootstrap.connect(host, port).sync();
}
public void send(String message) {
ByteBuf byteBuf = Unpooled.copiedBuffer(message, Charset.forName("UTF-8"));
channelFuture.channel().writeAndFlush(byteBuf);
}
public void close() {
workerGroup.shutdownGracefully();
}
// 自定义编码器
private static class MyEncoder extends MessageToByteEncoder<String> {
@Override
protected void encode(ChannelHandlerContext ctx, String msg, ByteBuf out) throws Exception {
out.writeInt(msg.length());
out.writeBytes(msg.getBytes());
}
}
// 自定义解码器
private static class MyDecoder extends ByteToMessageDecoder {
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List<Object> out) throws Exception {
if (in.readableBytes() >= 4) {
int length = in.readInt();
if (in.readableBytes() < length) {
in.resetReaderIndex();
return;
}
byte[] bytes = new byte[length];
in.readBytes(bytes);
String message = new String(bytes, Charset.forName("UTF-8"));
out.add(message);
}
}
}
// 自定义处理器
private static class MyHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("收到服务器消息:" + msg);
}
}
}
```
3. 在Spring Boot的启动类中创建AioQuickClient实例并连接服务器:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
AioQuickClient client = new AioQuickClient("127.0.0.1", 8888);
try {
client.connect();
} catch (InterruptedException e) {
e.printStackTrace();
}
client.send("Hello, server!");
client.close();
}
}
```
在以上示例中,我们使用了Netty的自定义编码器、解码器和处理器来处理数据的编解码和业务逻辑。你可以根据自己的需求进行相应的修改。
相关推荐
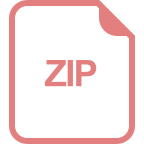
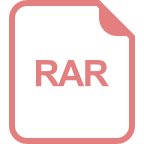














