vue3+ts怎么从父组件调用子组件的方法
时间: 2024-12-12 12:13:07 浏览: 30
在 Vue3 中使用 TypeScript,如果你想要从父组件调用子组件的方法,你可以通过 `ref`、`setup` 函数或者 prop 的自定义事件 (`v-on` 或者 `emitter`) 来实现。这里提供一种常见的做法:
1. **props 和 emit 传递方法**:
父组件中,可以将子组件需要的方法作为 prop 传给子组件:
```typescript
<template>
<child-component :myMethod="parentMethod" />
</template>
<script lang="ts">
import { Component, Prop } from 'vue';
import ChildComponent from './ChildComponent.vue';
@Component
export default class ParentComponent extends Vue {
parentMethod = () => {
// 父组件执行的操作
};
methods: {
callChildMethod() {
this.$refs.childComponent.myMethod();
}
}
}
</script>
```
然后在子组件中,接收这个方法并调用:
```typescript
<template>
<button @click="$emit('invokeParentMethod')">触发父方法</button>
</template>
<script lang="ts">
import { Component, Emit } from 'vue';
export default class ChildComponent extends Vue {
@Emit('invokeParentMethod') invokeParentMethod() {
this.parentMethod(); // 调用父组件的方法
}
}
</script>
```
2. **通过 ref 实现**
如果你想直接操作子组件实例,可以在父组件里创建一个 ref 引用子组件,并调用其方法:
```typescript
<template>
<child-component ref="childComponent" />
</template>
<script lang="ts">
import { Component, Ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
@Component
export default class ParentComponent extends Vue {
private childComponentRef: Ref<typeof ChildComponent>;
setup() {
this.childComponentRef = ref(null);
onMounted(() => {
if (this.childComponentRef.value) {
this.childComponentRef.value.myMethod();
}
});
}
mounted() {
// 初始化后设置 ref
this.childComponentRef = ref(this.$refs.childComponent);
}
}
</script>
```
记得在实际项目中,要处理可能出现的空引用异常(`this.childComponentRef.value`可能为空)。
阅读全文
相关推荐
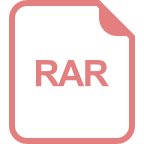
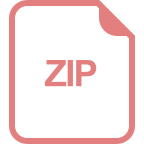
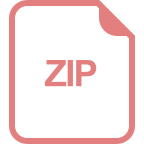















