shell编程中的if else
时间: 2023-11-08 18:05:03 浏览: 59
在shell编程中,if else语句用于根据条件的结果执行不同的代码块。它的基本语法如下:
```shell
if [ condition ]
then
# 如果条件成立,则执行这里的代码块
else
# 如果条件不成立,则执行这里的代码块
fi
```
其中,`condition`是一个表达式,可以使用比较运算符(如`-eq`、`-ne`、`-lt`、`-gt`、`-le`、`-ge`)或者逻辑运算符(如`-a`、`-o`、`!`)来构建条件。
下面是一个简单的例子,判断一个数字是否大于10,并输出相应的结果:
```shell
#!/bin/bash
number=15
if [ $number -gt 10 ]
then
echo "The number is greater than 10"
else
echo "The number is not greater than 10"
fi
```
输出结果为:"The number is greater than 10"。
相关问题
shell编程的if语句
以下是shell编程中if语句的几种用法:
1. 单分支结构:
```shell
if <条件表达式>; then
指令序列
fi
```
2. 双分支结构:
```shell
if <条件表达式>; then
指令序列1
else
指令序列2
fi
```
3. 多分支结构:
```shell
if <条件表达式1>; then
指令序列1
elif <条件表达式2>; then
指令序列2
elif <条件表达式3>; then
指令序列3
else
指令序列n
fi
```
4. 嵌套if语句:
```shell
if <条件表达式1>; then
指令序列1
if <条件表达式2>; then
指令序列2
else
指令序列3
fi
else
指令序列4
fi
```
以上是shell编程中if语句的几种常见用法,可以根据具体需求选择适合的结构来实现相应的逻辑判断和执行。
shell if else
在 shell 编程中,if-else 语句用于根据特定条件执行不同的代码块。if 语句测试一个条件是否为 true,如果是,则执行一些语句;否则,执行另外一些语句。else 语句用于在 if 语句的条件不为 true 时执行一些代码。
if-else 语句的语法如下:
```
if [ condition ]
then
# do something
else
# do something else
fi
```
其中,`condition` 是要测试的条件,可以是字符串、数值、文件是否存在等。
示例代码:
```
#!/bin/bash
count=10
if [ $count -eq 10 ]
then
echo "Count is 10"
else
echo "Count is not 10"
fi
```
在上面的示例中,我们使用 if-else 语句来测试变量 `count` 是否等于 `10`。如果等于 `10`,则输出 `Count is 10`;否则,输出 `Count is not 10`。
相关推荐
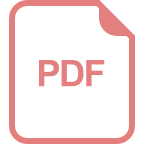
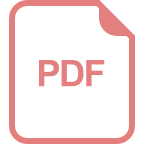
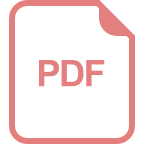












