用Java简单编写设计一个简单的学生选课系统,并可以完成添加、删除、修改、查询相关信息 。 说明: 可以设计以下类: 描述课程基本信息类(课程编号,课程名,学分 ); 描述学生基本信息类(学号,姓名,专业方向 ); 记录选课结果的类(课程,选课的学生,课程最多人数,当前人数 ); 测试类(设定或输入最多人数,创建课程对象,创建选课学生对象,创建选课对象,显示选课情况 )
时间: 2024-03-11 09:44:17 浏览: 19
好的,以下是一个简单的学生选课系统的Java代码:
Course.java
```java
public class Course {
private String courseID;
private String courseName;
private int credit;
public Course(String courseID, String courseName, int credit) {
this.courseID = courseID;
this.courseName = courseName;
this.credit = credit;
}
public String getCourseID() {
return courseID;
}
public String getCourseName() {
return courseName;
}
public int getCredit() {
return credit;
}
public void setCourseID(String courseID) {
this.courseID = courseID;
}
public void setCourseName(String courseName) {
this.courseName = courseName;
}
public void setCredit(int credit) {
this.credit = credit;
}
@Override
public String toString() {
return "课程编号:" + courseID + ", 课程名:" + courseName + ", 学分:" + credit;
}
}
```
Student.java
```java
public class Student {
private String studentID;
private String name;
private String major;
public Student(String studentID, String name, String major) {
this.studentID = studentID;
this.name = name;
this.major = major;
}
public String getStudentID() {
return studentID;
}
public String getName() {
return name;
}
public String getMajor() {
return major;
}
public void setStudentID(String studentID) {
this.studentID = studentID;
}
public void setName(String name) {
this.name = name;
}
public void setMajor(String major) {
this.major = major;
}
@Override
public String toString() {
return "学号:" + studentID + ", 姓名:" + name + ", 专业方向:" + major;
}
}
```
CourseSelection.java
```java
public class CourseSelection {
private Course course;
private Student student;
private int maxNum;
private int currentNum;
public CourseSelection(Course course, Student student, int maxNum, int currentNum) {
this.course = course;
this.student = student;
this.maxNum = maxNum;
this.currentNum = currentNum;
}
public Course getCourse() {
return course;
}
public Student getStudent() {
return student;
}
public int getMaxNum() {
return maxNum;
}
public int getCurrentNum() {
return currentNum;
}
public void setCourse(Course course) {
this.course = course;
}
public void setStudent(Student student) {
this.student = student;
}
public void setMaxNum(int maxNum) {
this.maxNum = maxNum;
}
public void setCurrentNum(int currentNum) {
this.currentNum = currentNum;
}
@Override
public String toString() {
return course.toString() + ", " + student.toString() + ", 最大人数:" + maxNum + ", 当前人数:" + currentNum;
}
}
```
Test.java
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class Test {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入课程最大人数:");
int maxNum = scanner.nextInt();
List<Course> courses = new ArrayList<>();
courses.add(new Course("001", "Java编程", 4));
courses.add(new Course("002", "Python编程", 3));
courses.add(new Course("003", "数据结构", 4));
courses.add(new Course("004", "操作系统", 3));
List<Student> students = new ArrayList<>();
students.add(new Student("1001", "张三", "计算机科学与技术"));
students.add(new Student("1002", "李四", "软件工程"));
students.add(new Student("1003", "王五", "信息安全"));
List<CourseSelection> selections = new ArrayList<>();
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 添加选课信息");
System.out.println("2. 删除选课信息");
System.out.println("3. 修改选课信息");
System.out.println("4. 查询选课信息");
System.out.println("0. 退出程序");
int choice = scanner.nextInt();
switch (choice) {
case 0:
System.out.println("程序已退出!");
return;
case 1:
System.out.println("请输入课程编号:");
String courseID = scanner.next();
Course course = null;
for (Course c : courses) {
if (c.getCourseID().equals(courseID)) {
course = c;
break;
}
}
if (course == null) {
System.out.println("没有找到编号为" + courseID + "的课程!");
break;
}
System.out.println("请输入学生学号:");
String studentID = scanner.next();
Student student = null;
for (Student s : students) {
if (s.getStudentID().equals(studentID)) {
student = s;
break;
}
}
if (student == null) {
System.out.println("没有找到学号为" + studentID + "的学生!");
break;
}
CourseSelection selection = new CourseSelection(course, student, maxNum, 1);
selections.add(selection);
System.out.println("添加选课信息成功!");
break;
case 2:
System.out.println("请输入课程编号:");
courseID = scanner.next();
studentID = scanner.next();
for (CourseSelection s : selections) {
if (s.getCourse().getCourseID().equals(courseID) && s.getStudent().getStudentID().equals(studentID)) {
selections.remove(s);
System.out.println("删除选课信息成功!");
break;
}
}
break;
case 3:
System.out.println("请输入课程编号和学生学号:");
courseID = scanner.next();
studentID = scanner.next();
for (CourseSelection s : selections) {
if (s.getCourse().getCourseID().equals(courseID) && s.getStudent().getStudentID().equals(studentID)) {
System.out.println("请输入最大人数和当前人数:");
int newMaxNum = scanner.nextInt();
int newCurrentNum = scanner.nextInt();
s.setMaxNum(newMaxNum);
s.setCurrentNum(newCurrentNum);
System.out.println("修改选课信息成功!");
break;
}
}
break;
case 4:
if (selections.size() == 0) {
System.out.println("没有任何选课信息!");
break;
}
System.out.println("课程编号\t课程名\t学分\t学生学号\t学生姓名\t专业方向\t最大人数\t当前人数");
for (CourseSelection s : selections) {
System.out.println(s.getCourse().getCourseID() + "\t" + s.getCourse().getCourseName() + "\t" + s.getCourse().getCredit() + "\t" +
s.getStudent().getStudentID() + "\t" + s.getStudent().getName() + "\t" + s.getStudent().getMajor() + "\t" +
s.getMaxNum() + "\t" + s.getCurrentNum());
}
break;
default:
System.out.println("输入有误,请重新选择!");
break;
}
}
}
}
```
这个简单的学生选课系统包含了课程、学生和选课结果三个类,以及一个测试类。在测试类中,我们创建了一些课程和学生的实例,并通过一个循环来执行添加、删除、修改和查询选课信息的操作。在添加选课信息时,我们需要输入课程编号和学生学号,系统会检查是否存在相应的课程和学生实例,如果存在,则创建一个选课结果的实例并添加到选课结果列表中。在删除和修改选课信息时,我们同样需要输入课程编号和学生学号,系统会遍历选课结果列表,找到对应的选课结果实例并执行删除或修改操作。在查询选课信息时,系统会输出所有的选课结果实例。
相关推荐
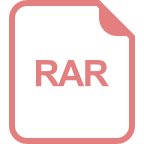
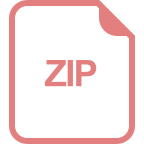
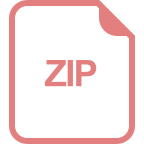














