opencv修改普通照片为证件照python
时间: 2023-10-06 12:10:57 浏览: 178
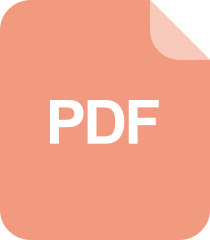
python opencv实现证件照换底功能
将普通照片修改为证件照,需要满足一些要求,如背景必须为纯色、人物面部不能有遮挡、尺寸及比例必须符合规定等。下面是使用OpenCV库实现将普通照片修改为证件照的步骤:
1. 读取原始照片,将其转化为灰度图像。
```python
import cv2
img = cv2.imread('original.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 使用人脸检测算法(如Haar级联分类器)检测人脸,并裁剪出人脸部分图像。
```python
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
if len(faces) > 0:
(x, y, w, h) = faces[0]
face = img[y:y+h, x:x+w]
```
3. 将人脸部分图像调整为标准证件照的尺寸(如国内标准为3.3cm×4.8cm)。
```python
resized_face = cv2.resize(face, (300, 430), interpolation=cv2.INTER_AREA)
```
4. 将调整后的人脸部分图像覆盖在一个纯色背景图像上,得到证件照。
```python
background = np.zeros((600, 600, 3), np.uint8)
background[:, :, :] = (255, 255, 255)
bg_h, bg_w, _ = background.shape
face_h, face_w, _ = resized_face.shape
offset_x, offset_y = (bg_w - face_w) // 2, (bg_h - face_h) // 2
background[offset_y:offset_y+face_h, offset_x:offset_x+face_w] = resized_face
cv2.imwrite('id_card.jpg', background)
```
这样就可以将普通照片修改为符合标准的证件照了。需要注意的是,上述代码中的参数(如人脸检测算法、尺寸、背景颜色等)需要根据实际情况进行调整。
阅读全文
相关推荐
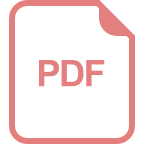


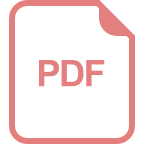
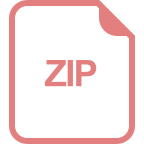
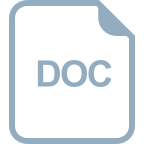
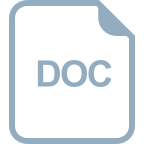





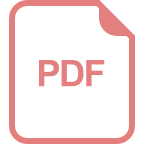
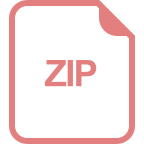