C++ 实现https请求
时间: 2023-07-27 19:06:28 浏览: 96
要在C++中实现HTTPS请求,可以使用第三方库,例如CURL或Boost.Beast。
使用CURL的示例代码如下:
```cpp
#include <iostream>
#include <curl/curl.h>
int main()
{
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "https://www.example.com");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
res = curl_easy_perform(curl);
if (res != CURLE_OK)
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
```
这个示例代码使用libcurl库,它提供了一个易于使用的API,用于发送HTTP或HTTPS请求。在使用libcurl时,需要先调用`curl_global_init()`初始化库,然后创建一个CURL对象,并设置请求的URL和其他选项。最后,调用`curl_easy_perform()`函数发送请求并接收响应。如果请求成功,该函数返回CURLE_OK,否则返回一个错误代码。
请注意,HTTPS请求需要使用SSL / TLS协议进行加密。为了支持HTTPS请求,需要在编译时启用libcurl的SSL支持,并提供SSL库和证书文件。在Unix / Linux系统上,可以使用OpenSSL库。在Windows系统上,可以使用Schlumberger Cryptographic Service Provider或Microsoft CryptoAPI等。
如果使用Boost.Beast库发送HTTPS请求,示例代码如下:
```cpp
#include <iostream>
#include <boost/beast/core.hpp>
#include <boost/beast/ssl.hpp>
#include <boost/beast/http.hpp>
#include <boost/asio/connect.hpp>
#include <boost/asio/ip/tcp.hpp>
namespace beast = boost::beast;
namespace http = beast::http;
namespace net = boost::asio;
namespace ssl = net::ssl;
using tcp = net::ip::tcp;
int main()
{
net::io_context ioc;
ssl::context ctx(ssl::context::tlsv12_client);
tcp::resolver resolver(ioc);
beast::ssl_stream<beast::tcp_stream> stream(ioc, ctx);
auto const results = resolver.resolve("www.example.com", "443");
beast::get_lowest_layer(stream).connect(results);
stream.handshake(ssl::stream_base::client);
http::request<http::string_body> req{http::verb::get, "/", 11};
req.set(http::field::host, "www.example.com");
req.set(http::field::user_agent, "Beast");
http::write(stream, req);
beast::flat_buffer buffer;
http::response<http::dynamic_body> res;
http::read(stream, buffer, res);
std::cout << res << std::endl;
beast::error_code ec;
stream.shutdown(ec);
if (ec == net::error::eof)
ec = {};
if (ec)
std::cerr << "Error: " << ec.message() << std::endl;
return 0;
}
```
这个示例代码使用Boost.Beast库,它是一个现代C ++网络库,提供了HTTP和WebSocket支持。示例代码创建了一个SSL上下文对象,并使用DNS解析器解析主机名和端口号。然后,它创建一个SSL流对象,并与服务器进行握手。接下来,示例代码构造一个HTTP请求对象,并将其写入流中。最后,它读取服务器的响应并打印到标准输出中。
请注意,Boost.Beast库需要Boost.Asio库和OpenSSL库的支持。在使用Boost.Beast时,需要在编译时链接这些库。
相关推荐
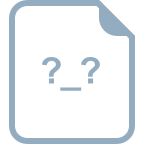














