python怎么使用xpath爬取京东商品
时间: 2024-01-02 16:03:42 浏览: 166
要使用Python和XPath来爬取京东商品,你可以按照以下步骤进行操作:
1. 首先,导入相应的库,包括selenium库和xpath库。Selenium库用于模拟浏览器行为,而XPath库用于解析网页的HTML结构。
2. 接下来,使用Selenium库打开京东网站,并输入你想要查询的商品关键词,发送请求。
3. 等待网页加载完成后,通过XPath表达式来选取商品信息的节点。你可以使用Chrome浏览器的开发者工具来查看网页的HTML结构,以确定XPath表达式。
4. 使用XPath库提取商品的相关信息,比如名称、价格、评价等。
5. 如果还有下一页的商品需要爬取,可以使用循环来翻页。判断是否应该点击下一页的条件可以通过检查网页中是否存在“下一页”按钮的XPath来判断。
6. 最后,将爬取到的商品信息保存到文件或者数据库中,或者进行其他后续的数据分析处理。
需要注意的是,在爬取京东商品时,京东的网页有一部分是通过动态加载的,所以需要使用Selenium库来模拟浏览器的滚动操作,等待所有数据加载完成后再进行解析。
以上就是使用Python和XPath爬取京东商品的基本步骤。希望对你有帮助!
相关问题
python网页爬虫爬取京东手机详情数据——Xpath数据解析
Python 网页爬虫利用 XPath 进行京东手机详情数据的爬取,是因为 XPath 是一种用于在 XML 或 HTML 文档中选择元素的语言,非常适合处理结构化的网页内容。下面是简单的步骤:
1. **安装库**:首先,你需要安装 Python 的爬虫库,如 `requests`(用于发送 HTTP 请求)和 `BeautifulSoup` 或 `lxml`(用于解析 HTML 结构)。
```bash
pip install requests beautifulsoup4
# 或者使用 lxml 提高性能
pip install lxml
```
2. **发送请求**:使用 `requests.get` 发送 GET 请求到京东商品详情页面的 URL。
```python
import requests
url = 'https://item.jd.com/<商品ID>.html'
response = requests.get(url)
```
3. **解析HTML**:使用 BeautifulSoup 解析返回的 HTML 内容,找到包含所需数据的部分。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'lxml')
xpath_query = '//*[@id="main"]/div[@class="sku-item"]' # 使用适合的XPath表达式
data_elements = soup.select(xpath_query) # 找到所有匹配的数据节点
```
4. **提取数据**:遍历找到的数据元素,使用 `.text`、`.get('attr')` 等方法获取具体的属性值。例如,获取手机名称、价格等信息。
```python
for element in data_elements:
title = element.find('span', {'class': 'p-name'}).text
price = element.find('i', {'class': 'p-price'}).get('title')
print(f"标题: {title}, 价格: {price}")
```
5. **保存数据**:如果你想将数据持久化,可以将其写入文件、数据库或 JSON 文件中。
python使用scrapy爬取京东多页数据并且将数据传入数据库,然后用pyecharts做数据可视化
好的,这是一个比较复杂的问题,需要分几个步骤来完成。
1. 安装和配置Scrapy
首先,你需要安装Scrapy。你可以在终端中使用以下命令安装:
```
pip install scrapy
```
安装完成后,你需要在Scrapy项目中配置以下文件:`settings.py`、`items.py`、`pipelines.py`。
其中,`settings.py`文件中需要配置一些参数,如下所示:
```
BOT_NAME = 'jd'
SPIDER_MODULES = ['jd.spiders']
NEWSPIDER_MODULE = 'jd.spiders'
ROBOTSTXT_OBEY = False
ITEM_PIPELINES = {
'jd.pipelines.JdPipeline': 300,
}
FEED_EXPORT_ENCODING = 'utf-8'
```
`items.py`文件中定义了我们要抓取的数据字段,如下所示:
```
import scrapy
class JdItem(scrapy.Item):
title = scrapy.Field()
price = scrapy.Field()
comment = scrapy.Field()
shop = scrapy.Field()
```
`pipelines.py`文件中我们可以对抓取到的数据进行处理,然后将其存入数据库中,如下所示:
```
import pymysql
class JdPipeline(object):
def __init__(self):
self.connect = pymysql.connect(
host='localhost',
port=3306,
db='jd',
user='root',
passwd='123456',
charset='utf8',
use_unicode=True)
self.cursor = self.connect.cursor()
def process_item(self, item, spider):
self.cursor.execute(
"""insert into jd_goods(title, price, comment, shop)
value (%s, %s, %s, %s)""",
(item['title'], item['price'], item['comment'], item['shop']))
self.connect.commit()
return item
```
2. 编写Scrapy爬虫
接下来,你需要编写一个Scrapy爬虫来爬取京东商品数据。这里以爬取“手机”关键词的商品数据为例,爬取多页数据。
```
import scrapy
from jd.items import JdItem
class JdSpider(scrapy.Spider):
name = 'jd'
allowed_domains = ['jd.com']
start_urls = ['https://search.jd.com/Search?keyword=手机&enc=utf-8']
def parse(self, response):
goods_list = response.xpath('//ul[@class="gl-warp clearfix"]/li')
for goods in goods_list:
item = JdItem()
item['title'] = goods.xpath('div[@class="gl-i-wrap"]/div[@class="p-name"]/a/em/text()').extract_first()
item['price'] = goods.xpath('div[@class="gl-i-wrap"]/div[@class="p-price"]/strong/i/text()').extract_first()
item['comment'] = goods.xpath('div[@class="gl-i-wrap"]/div[@class="p-commit"]/strong/a/text()').extract_first()
item['shop'] = goods.xpath('div[@class="gl-i-wrap"]/div[@class="p-shop"]/span/a/text()').extract_first()
yield item
# 翻页
next_page = response.xpath('//a[@class="pn-next"]/@href')
if next_page:
url = response.urljoin(next_page.extract_first())
yield scrapy.Request(url, callback=self.parse)
```
在命令行中输入以下命令运行Scrapy爬虫:
```
scrapy crawl jd
```
3. 将数据可视化
最后,你需要使用Pyecharts将爬取到的数据进行可视化。这里以柱状图为例,代码如下所示:
```
import pymysql
from pyecharts import options as opts
from pyecharts.charts import Bar
connect = pymysql.connect(
host='localhost',
port=3306,
db='jd',
user='root',
passwd='123456',
charset='utf8',
use_unicode=True)
cursor = connect.cursor()
cursor.execute("""SELECT shop, COUNT(*) FROM jd_goods GROUP BY shop""")
data = cursor.fetchall()
bar = (
Bar()
.add_xaxis([i[0] for i in data])
.add_yaxis("商品数量", [i[1] for i in data])
.set_global_opts(title_opts=opts.TitleOpts(title="京东手机商品店铺分布"))
)
bar.render("jd.html")
```
最后,你可以在浏览器中打开`jd.html`文件,查看生成的柱状图。
阅读全文
相关推荐
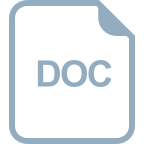
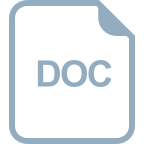
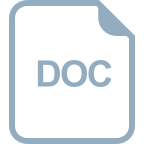
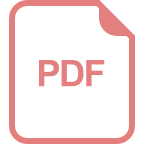
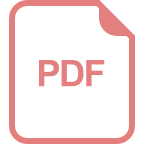
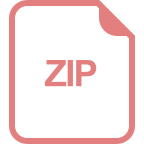


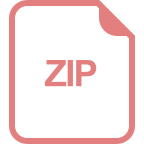
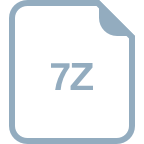
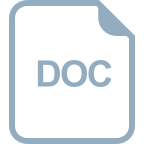





