Java创建radio控件
时间: 2024-12-31 22:47:26 浏览: 6
在Java中,创建Radio Button控件通常是在Swing或JavaFX GUI库中操作。以下是使用Swing库创建简单单选按钮的一般步骤:
1. 导入javax.swing包:
```java
import javax.swing.*;
```
2. 创建JRadioButton实例并添加到容器(如JPanel)中:
```java
JRadioButton radioButton1 = new JRadioButton("Option 1");
JRadioButton radioButton2 = new JRadioButton("Option 2");
// 将按钮添加到面板上
JPanel panel = new JPanel();
panel.add(radioButton1);
panel.add(radioButton2);
```
如果你想设置默认选择,可以使用`isSelected()`和`setEnabled()`方法:
```java
radiobutton1.setSelected(true); // 设置第一个选项为默认选中
// 或者,通过监听ButtonGroup来管理一组互斥的单选按钮
ButtonGroup group = new ButtonGroup(); // 创建一个ButtonGroup
group.add(radioButton1); // 添加按钮到组中
```
相关问题
请帮我根据以下要求写出具体代码 Android中创建一个 Activity 名为:Activity+学号后 3 位.java,实现一道防疫知识问答题。 a) 要求使用文本框输入答案,单击按钮判断对错,并提示对错或要求使用单选框选择单项选择答案;使用复选框选择多选答案),并将结果存储在 xml 文件中,文件名“姓名的拼音简称.xml”。(30 分) b) 使用合适的布局,要求使用背景图片(或自定义的 drawable 对象 xml 文件、或自定颜色)。(10 分) c) 使用合适的控件,控件属性要求:
好的,以下是代码实现:
```java
public class ActivityXXX extends AppCompatActivity {
private EditText answerEditText;
private RadioGroup answerRadioGroup;
private CheckBox answerCheckBox1;
private CheckBox answerCheckBox2;
private CheckBox answerCheckBox3;
private Button submitButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_xxx);
answerEditText = findViewById(R.id.answer_edit_text);
answerRadioGroup = findViewById(R.id.answer_radio_group);
answerCheckBox1 = findViewById(R.id.answer_check_box1);
answerCheckBox2 = findViewById(R.id.answer_check_box2);
answerCheckBox3 = findViewById(R.id.answer_check_box3);
submitButton = findViewById(R.id.submit_button);
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String answer = answerEditText.getText().toString();
boolean isCorrect = checkAnswer(answer);
if (isCorrect) {
Toast.makeText(ActivityXXX.this, "回答正确!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(ActivityXXX.this, "回答错误!", Toast.LENGTH_SHORT).show();
}
}
});
}
private boolean checkAnswer(String answer) {
// 进行答案判断,返回是否正确的布尔值
}
public void onRadioButtonClicked(View view) {
// 处理单选框选择答案的逻辑
}
public void onCheckBoxClicked(View view) {
// 处理复选框选择答案的逻辑
}
// 将结果存储在 xml 文件中
private void saveResultToXml(String fileName) {
// 实现将结果存储在 xml 文件中的代码
}
}
```
其中,`activity_xxx.xml` 文件中应该包括输入答案的文本框、单选框、复选框、提交按钮等控件,并设置对应的 ID 和布局属性。保存结果至 xml 文件可使用 Android 提供的 XML 解析和生成库完成。在 `AndroidManifest.xml` 中注册该 Activity。
android radio button
Android中的RadioButton是一种用于单选的可选控件。它通常与RadioGroup一起使用,RadioGroup是一种容器,它只允许用户选择一个选项。
以下是创建RadioButton的步骤:
1. 在XML布局文件中添加RadioGroup控件和若干RadioButton控件。
2. 为每个RadioButton控件设置唯一的ID。
3. 在Java代码中获取RadioGroup控件的引用,并设置它的OnCheckedChangeListener。
4. 在OnCheckedChangeListener中处理RadioButton的选择事件。
以下是一个示例:
```xml
<RadioGroup
android:id="@+id/radio_group"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RadioButton
android:id="@+id/radio_button_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Option 1" />
<RadioButton
android:id="@+id/radio_button_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Option 2" />
</RadioGroup>
```
```java
RadioGroup radioGroup = findViewById(R.id.radio_group);
radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup radioGroup, int checkedId) {
if (checkedId == R.id.radio_button_1) {
// Option 1 selected
} else if (checkedId == R.id.radio_button_2) {
// Option 2 selected
}
}
});
```
阅读全文
相关推荐






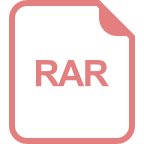









