用JAVA中的输入输出流写下面的程序,该程序将100个随机生成的(0到1000)整数写入文件,每个整数以“#”分隔。然后,从该文件中读取这些整数,并计算最大值、最小值和平均值并输出。
时间: 2024-12-23 20:12:36 浏览: 0
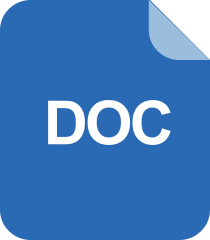
实验四 字符串和输入输出流的实验(1).doc

在Java中,你可以使用`FileOutputStream`和`BufferedWriter`来写入数据,`Scanner`和`FileReader`来读取数据,以及`Random`生成随机数。下面是实现这个功能的一个简单示例:
```java
import java.io.*;
import java.util.Random;
public class RandomIntegerIO {
public static void main(String[] args) {
try {
// 写入操作
File file = new File("random_numbers.txt");
FileOutputStream fos = new FileOutputStream(file);
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(fos));
Random random = new Random();
int count = 0;
while (count < 100) {
int number = random.nextInt(1001); // 生成0到1000的随机整数
bw.write(Integer.toString(number)); // 将整数转换成字符串并写入
if (count != 99) { // 除了最后一个,每行结尾加上#
bw.newLine();
bw.write("#");
}
count++;
}
bw.close(); // 关闭缓冲 writer
fos.close(); // 关闭文件输出流
// 读取操作
Scanner sc = new Scanner(new FileReader(file));
String line;
int sum = 0, max = Integer.MIN_VALUE, min = Integer.MAX_VALUE;
while ((line = sc.nextLine()) != null) {
String[] numbers = line.split("#"); // 按"#"分割字符串
for (String num : numbers) {
int temp = Integer.parseInt(num);
sum += temp;
if (temp > max) {
max = temp;
} else if (temp < min) {
min = temp;
}
}
}
sc.close();
double average = (double) sum / 100; // 计算平均值
System.out.println("最大值: " + max);
System.out.println("最小值: " + min);
System.out.println("平均值: " + average);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
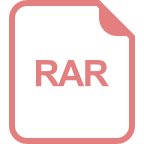
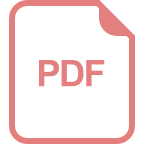



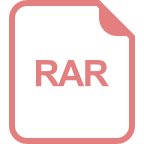
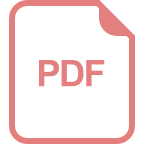
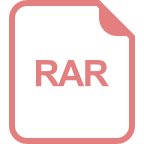
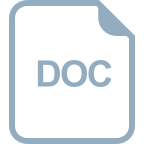
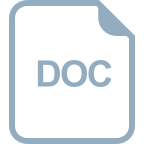
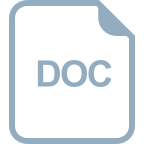
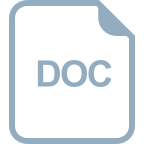
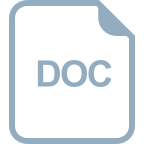
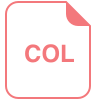
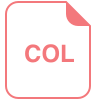


