如何使用HttpURLConnection返回api获取到的response
时间: 2024-04-18 09:33:25 浏览: 11
要使用 `HttpURLConnection` 获取 API 的响应,可以按照以下步骤进行操作:
1. 创建 `URL` 对象,指定要请求的 API 的 URL:
```java
URL url = new URL("https://api.example.com/endpoint");
```
2. 打开连接并设置请求方法为 GET(或其他适当的方法):
```java
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
```
3. 如果需要设置请求头,可以使用 `setRequestProperty` 方法添加请求头参数:
```java
connection.setRequestProperty("Content-Type", "application/json");
```
4. 发送请求,并获取响应状态码:
```java
int responseCode = connection.getResponseCode();
```
5. 根据响应状态码判断请求是否成功:
```java
if (responseCode == HttpURLConnection.HTTP_OK) {
// 请求成功,读取响应内容
InputStream inputStream = connection.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// 处理响应内容
System.out.println(response.toString());
} else {
// 请求失败,根据需要处理错误
System.out.println("请求失败,错误码:" + responseCode);
}
```
6. 最后,记得关闭连接:
```java
connection.disconnect();
```
以上是使用 `HttpURLConnection` 获取 API 响应的基本步骤。你可以根据需要调整和扩展代码来适应不同的场景和要求。
相关推荐
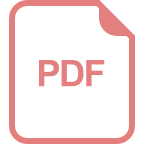














