不下载nltk库,进行英文词频统计排除停用词并输出排行前十词汇
时间: 2024-11-27 14:23:13 浏览: 29
如果你不想直接使用Python的NLTK库来进行英文词频统计并排除停用词,你可以手动编写一些代码来完成这个任务。首先,你需要一个英文文本数据源,然后按照以下步骤操作:
1. **读取文本**:
使用`open()`函数打开文件,并读取其中的内容。
```python
with open('your_text_file.txt', 'r') as file:
text = file.read().lower()
```
2. **分词**:
使用正则表达式将文本分割成单词列表,忽略标点符号和其他非字母字符。
```python
import re
words = re.findall(r'\b\w+\b', text)
```
3. **停用词处理**:
制作一个停用词列表,包括常见的英语停用词。NLTK库提供了一个预定义的停用词列表,如果没有下载,你可以手动创建一个,比如从网上查找并添加到一个set中。
```python
# 如果没用NLTK,手动创建停用词集合
stop_words = {'the', 'a', 'an', 'and', ...} # 添加更多常见停用词
filtered_words = [word for word in words if word not in stop_words]
```
4. **词频统计**:
使用`collections.Counter`来计算每个单词出现的频率。
```python
from collections import Counter
word_counts = Counter(filtered_words)
```
5. **排序和输出**:
获取词频最高的前十个词汇及其频率。
```python
top_10 = word_counts.most_common(10)
for word, freq in top_10:
print(f'{word}: {freq}')
```
阅读全文
相关推荐
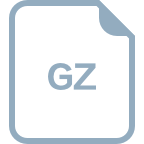

















