本题要求实现一个函数用顺序查找法,从前向后查找元素出现的位置及查询次数c语言。
时间: 2024-09-19 09:02:44 浏览: 35
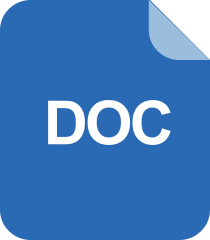
数据结构C语言版章节练习题(1-6章).doc
在C语言中,你可以创建一个函数来实现顺序查找算法。这种方法遍历数组,从第一个元素开始逐个比较,直到找到目标元素或搜索完整个数组。这里是一个简单的示例函数,它接收一个整数数组、数组长度以及一个目标值作为输入,并返回目标值在数组中的索引及其出现的次数:
```c
#include <stdio.h>
// 函数声明,返回值为 int 和 int 对应位置和计数
typedef struct {
int position;
int count;
} SearchResult;
SearchResult sequential_search(int arr[], int len, int target) {
SearchResult result = { -1, 0 };
for (int i = 0; i < len; ++i) {
if (arr[i] == target) {
// 如果找到目标值,更新位置并增加计数
result.position = i;
++result.count;
}
}
return result;
}
int main() {
int array[] = {1, 2, 3, 4, 5, 2, 6};
int len = sizeof(array) / sizeof(array[0]);
int target = 2;
SearchResult search_result = sequential_search(array, len, target);
if (search_result.position != -1) {
printf("Element %d found at index %d and appears %d times.\n",
target, search_result.position, search_result.count);
} else {
printf("Element not found in the array.\n");
}
return 0;
}
```
在这个例子中,`sequential_search`函数会查找数组中给定的目标值,如果找到,则返回一个结构体,包含元素的位置和出现次数;如果没有找到,则返回一个表示未找到的结果。
阅读全文
相关推荐
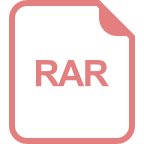
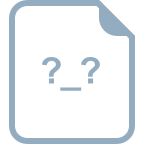

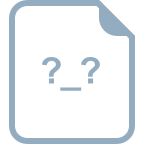
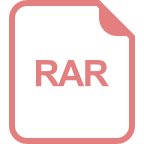
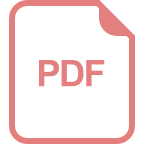
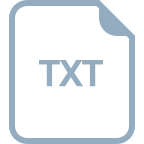
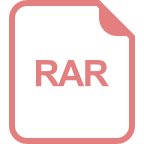
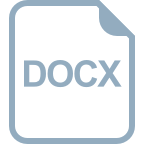
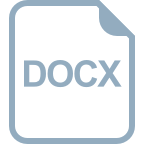
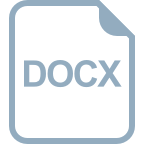
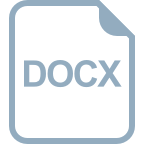
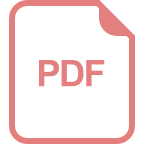
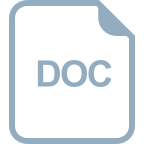
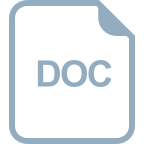
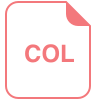
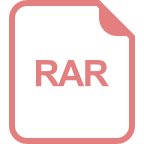