java应用程序 bresenham算法画向内凹陷的菱形
时间: 2023-11-18 07:44:42 浏览: 77
要使用Bresenham算法画一个向内凹陷的菱形,你可以按照以下步骤进行:
1. 首先,确定菱形的中心点和大小。假设菱形的中心点为(cx, cy),边长为size。
2. 然后,通过绘制线段来生成菱形的四条边。你可以使用Bresenham算法来绘制直线段。
3. 对于每条边,你需要计算起点和终点坐标。可以根据菱形的中心点和大小来计算。
4. 最后,调用Bresenham算法来绘制每条边。
以下是一个使用Java编写的示例代码:
```java
public class BresenhamDiamond {
public static void drawDiamond(int cx, int cy, int size) {
// 计算起点和终点坐标
int x = cx;
int y = cy - size/2;
int xEnd = cx + size/2;
int yEnd = cy;
// 绘制上半部分边
drawLine(x, y, xEnd, yEnd);
// 计算起点和终点坐标
x = cx + size/2;
y = cy;
xEnd = cx;
yEnd = cy + size/2;
// 绘制右半部分边
drawLine(x, y, xEnd, yEnd);
// 计算起点和终点坐标
x = cx;
y = cy + size/2;
xEnd = cx - size/2;
yEnd = cy;
// 绘制下半部分边
drawLine(x, y, xEnd, yEnd);
// 计算起点和终点坐标
x = cx - size/2;
y = cy;
xEnd = cx;
yEnd = cy - size/2;
// 绘制左半部分边
drawLine(x, y, xEnd, yEnd);
}
public static void drawLine(int x0, int y0, int x1, int y1) {
// 使用Bresenham算法绘制直线段
// 这里省略具体的实现
// 输出绘制的直线段
System.out.println("绘制直线段:(" + x0 + ", " + y0 + ") -> (" + x1 + ", " + y1 + ")");
}
public static void main(String[] args) {
int centerX = 100; int centerY = 100;
int size = 50;
drawDiamond(centerX, centerY, size);
}
}
```
阅读全文
相关推荐




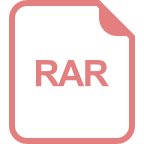
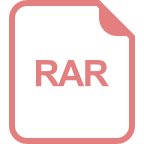





