sql语句操作hbase数据库
时间: 2023-06-28 08:05:42 浏览: 53
HBase是一个基于Hadoop的分布式非关系型数据库,它与传统的关系型数据库不同,没有使用SQL语言。但是,HBase提供了类似于SQL的查询语言HBase Shell,可以使用类似于SQL的语法(例如,SELECT、WHERE、AND、OR等)来查询HBase中的数据。
除了HBase Shell,还可以使用Java API或者REST API来操作HBase数据库。以下是使用Java API进行HBase数据库操作的示例代码:
```java
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.Delete;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.client.Table;
import org.apache.hadoop.hbase.util.Bytes;
public class HBaseDemo {
private static final String TABLE_NAME = "mytable";
private static final String COLUMN_FAMILY = "cf";
public static void main(String[] args) throws IOException {
// 创建HBase配置对象
org.apache.hadoop.conf.Configuration config = HBaseConfiguration.create();
// 创建HBase连接对象
Connection connection = ConnectionFactory.createConnection(config);
// 获取HBase表对象
Table table = connection.getTable(TableName.valueOf(TABLE_NAME));
// 插入数据
Put put = new Put(Bytes.toBytes("row1"));
put.addColumn(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes("col1"), Bytes.toBytes("value1"));
table.put(put);
// 查询数据
Get get = new Get(Bytes.toBytes("row1"));
Result result = table.get(get);
byte[] value = result.getValue(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes("col1"));
System.out.println("Value of col1: " + Bytes.toString(value));
// 扫描数据
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
List<String> rows = new ArrayList<>();
for (Result scanResult : scanner) {
byte[] row = scanResult.getRow();
rows.add(Bytes.toString(row));
}
System.out.println("Rows in table: " + rows);
// 删除数据
Delete delete = new Delete(Bytes.toBytes("row1"));
table.delete(delete);
// 关闭连接
table.close();
connection.close();
}
}
```
上述代码演示了如何使用Java API进行HBase数据库的数据插入、查询、扫描和删除操作。需要注意的是,这里使用的是HBase 1.x版本的Java API,如果使用其他版本的HBase,需要相应地更改API的包名和方法名。
相关推荐
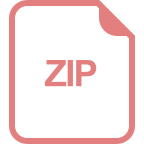
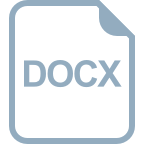














