通过断言判断一个数组为空则抛出自定义的异常
时间: 2024-09-25 08:10:25 浏览: 31
在Java中,如果你想通过断言判断一个数组是否为空,并在它为空时抛出自定义的异常,你可以这样做:
首先,你需要定义一个自定义的异常类,比如我们创建一个名为`CustomArrayException`的类,让它继承自`RuntimeException`:
```java
public class CustomArrayException extends RuntimeException {
public CustomArrayException(String message) {
super(message);
}
}
```
然后,在需要检查数组是否为空的地方,添加断言并处理异常:
```java
public void processArray(int[] array) {
assert array != null : "数组不能为空";
// 如果数组真的为空,这行代码将不会被执行
// 因为断言失败会导致抛出异常
if (array.length == 0) {
throw new CustomArrayException("数组长度为0");
}
// 正常的数组操作...
}
```
当调用`processArray`方法时,如果传入的是null数组,断言会失败,程序会立即抛出`CustomArrayException`异常,并显示指定的消息。
阅读全文
相关推荐
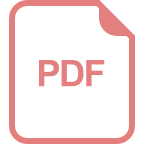
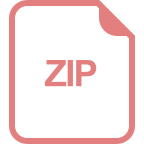
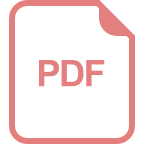
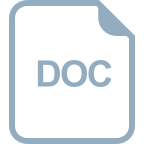
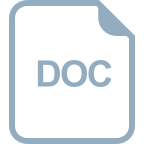
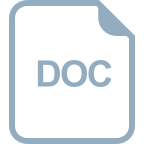
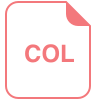
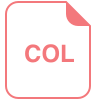
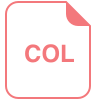
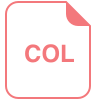
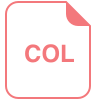
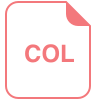
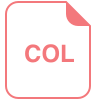
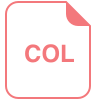
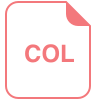
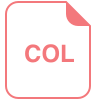
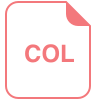
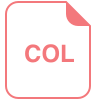
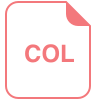