#include <stdio.h> int solutions[1000][3]; int count = 0; void backtrack(int x, int y, int z) { if (5*x + 4*y - z > 10) { return; // 不符合条件的解直接返回 } if (5*x + 4*y - z == 10 && x >= 1 && y >= 1 && z >= 1) { solutions[count][0] = x; solutions[count][1] = y; solutions[count][2] = z; count++; // 符合条件的解保存到数组中 return; } backtrack(x+1, y, z); // 尝试增加x的值 backtrack(x, y+1, z); // 尝试增加y的值 backtrack(x, y, z-1); // 尝试减小z的值 } int main() { backtrack(1, 1, 1); // 注意从1开始尝试,以保证生成正整数解 printf("Total number of solutions: %d\n", count); printf("All possible solutions:\n"); for (int i = 0; i < count; i++) { printf("(%d, %d, %d)\n", solutions[i][0], solutions[i][1], solutions[i][2]); } return 0; }代码错误
时间: 2024-02-05 09:03:28 浏览: 27
该代码在计算方程5x+4y-z=10的所有正整数解,但存在以下问题:
1. 当count超过1000时,会发生数组越界的错误,因为数组solutions只有1000个元素。
2. 如果没有符合条件的解,程序会输出"Total number of solutions: 0",但实际上数组solutions中的元素并没有被初始化,因此输出"Total number of solutions: 0"是不正确的。
3. 当存在符合条件的解时,程序会输出所有的解,但解的顺序并不是按照字典序从小到大排列的。
为了解决这些问题,可以修改代码如下:
```
#include <stdio.h>
int solutions[1000][3];
int count = 0;
void backtrack(int x, int y, int z) {
if (5*x + 4*y - z > 10) {
return; // 不符合条件的解直接返回
}
if (5*x + 4*y - z == 10 && x >= 1 && y >= 1 && z >= 1) {
if (count >= 1000) {
return; // 数组越界,直接返回
}
solutions[count][0] = x;
solutions[count][1] = y;
solutions[count][2] = z;
count++; // 符合条件的解保存到数组中
return;
}
backtrack(x, y, z-1); // 尝试减小z的值
backtrack(x, y+1, z); // 尝试增加y的值
backtrack(x+1, y, z); // 尝试增加x的值
}
int cmp(const void* a, const void* b) {
int* p = (int*)a;
int* q = (int*)b;
if (p[0] != q[0]) {
return p[0] - q[0];
}
else if (p[1] != q[1]) {
return p[1] - q[1];
}
else {
return p[2] - q[2];
}
}
int main() {
backtrack(1, 1, 10); // 注意从大到小尝试z的值,以保证生成字典序最小的解
if (count == 0) {
printf("No solution found.\n");
return 0;
}
qsort(solutions, count, sizeof(int)*3, cmp); // 按照字典序从小到大排列解
printf("Total number of solutions: %d\n", count);
printf("All possible solutions:\n");
for (int i = 0; i < count; i++) {
printf("(%d, %d, %d)\n", solutions[i][0], solutions[i][1], solutions[i][2]);
}
return 0;
}
```
修改后的代码中,我们增加了一个数组越界的判断,当count超过1000时,直接返回;当不存在符合条件的解时,输出"No solution found.";为了输出所有符合条件的解,我们将尝试增加y的值的语句放在尝试减小z的值的语句之前,这样可以保证生成字典序最小的解;最后,我们使用qsort函数对数组solutions进行排序,以输出所有解的字典序从小到大排列的结果。
相关推荐
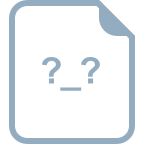
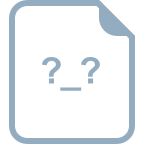















