pyflink的开发模板
时间: 2023-09-15 10:19:00 浏览: 91
PyFlink 提供了多种不同的开发模板,可供开发人员使用。以下是其中的一些模板:
1. Batch job 模板:
```python
from pyflink.dataset import ExecutionEnvironment
exec_env = ExecutionEnvironment.get_execution_environment()
input_path = "path/to/input"
output_path = "path/to/output"
data = exec_env.read_text_file(input_path)
result = data \
.map(lambda x: x.split(",")) \
.filter(lambda x: len(x) == 2) \
.map(lambda x: (x[0], int(x[1]))) \
.group_by(0) \
.sum(1)
result.write_as_csv(output_path, "\n", ",")
exec_env.execute("Batch Job Example")
```
2. Streaming job 模板:
```python
from pyflink.datastream import StreamExecutionEnvironment
from pyflink.datastream import TimeCharacteristic
env = StreamExecutionEnvironment.get_execution_environment()
env.set_stream_time_characteristic(TimeCharacteristic.EventTime)
input_path = "path/to/input"
data = env.read_text_file(input_path)
data = data \
.map(lambda x: x.split(",")) \
.filter(lambda x: len(x) == 2) \
.map(lambda x: (x[0], int(x[1]))) \
.assign_timestamps_and_watermarks(MyTimestampExtractor())
result = data \
.key_by(lambda x: x[0]) \
.time_window(Time.seconds(10)) \
.reduce(lambda x, y: (x[0], x[1] + y[1]))
result.print()
env.execute("Streaming Job Example")
```
3. Table job 模板:
```python
from pyflink.table import TableEnvironment
t_env = TableEnvironment.get_batch_environment()
input_path = "path/to/input"
output_path = "path/to/output"
t_env \
.read_csv(input_path, ['word', 'count'], field_delim=',') \
.where("count > 10") \
.group_by("word") \
.select("word, count(1) as count") \
.write_csv(output_path, field_delim=',')
t_env.execute("Table Job Example")
```
以上是 PyFlink 中的三种常用开发模板,可以根据实际情况进行调整和扩展。
阅读全文
相关推荐
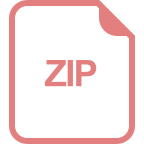
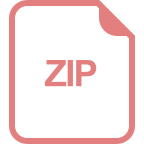
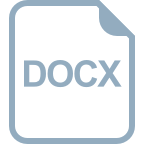
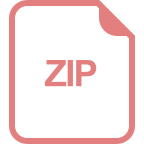
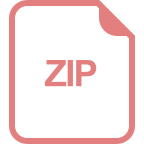
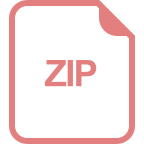
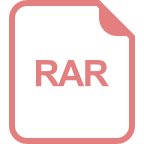
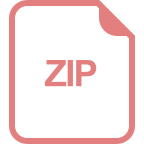
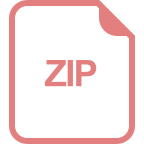
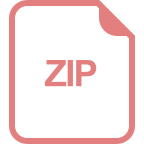
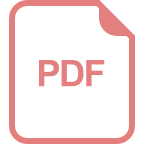
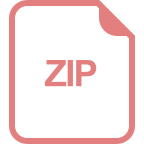
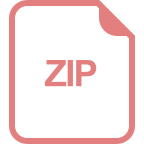