c++派生类求线段长度和中点坐标
时间: 2024-06-15 07:09:04 浏览: 121
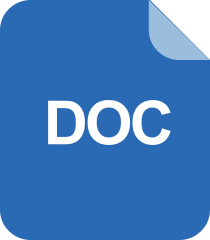
c++类的派生详细例子

在C++中,派生类可以通过继承基类的属性和方法,并且可以添加自己的属性和方法。如果你想在派生类中求线段长度和中点坐标,可以按照以下步骤进行:
1. 创建一个基类,例如名为"Line"的类,其中包含线段的起点和终点坐标。
2. 在基类中添加一个计算线段长度的方法,可以使用两点间的距离公式来计算。
3. 创建一个派生类,例如名为"DerivedLine"的类,继承自基类"Line"。
4. 在派生类中添加一个计算线段中点坐标的方法,可以通过取起点和终点坐标的平均值来计算。
5. 在主程序中创建一个派生类对象,调用该对象的方法来求线段长度和中点坐标。
下面是一个示例代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Line {
protected:
double x1, y1; // 起点坐标
double x2, y2; // 终点坐标
public:
Line(double x1, double y1, double x2, double y2) {
this->x1 = x1;
this->y1 = y1;
this->x2 = x2;
this->y2 = y2;
}
double calculateLength() {
double length = sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2));
return length;
}
};
class DerivedLine : public Line {
public:
DerivedLine(double x1, double y1, double x2, double y2) : Line(x1, y1, x2, y2) {}
void calculateMidPoint() {
double midX = (x1 + x2) / 2;
double midY = (y1 + y2) / 2;
cout << "中点坐标:(" << midX << ", " << midY << ")" << endl;
}
};
int main() {
double x1, y1, x2, y2;
cout << "请输入线段的起点坐标和终点坐标:" << endl;
cin >> x1 >> y1 >> x2 >> y2;
DerivedLine line(x1, y1, x2, y2);
double length = line.calculateLength();
cout << "线段长度:" << length << endl;
line.calculateMidPoint();
return 0;
}
```
阅读全文
相关推荐
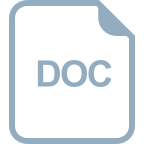
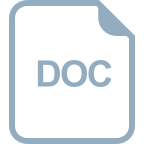
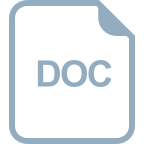
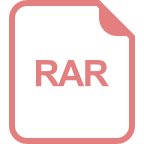
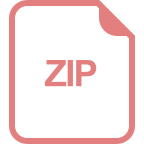
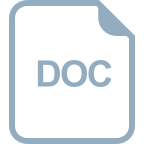
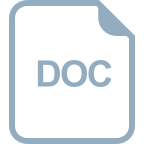
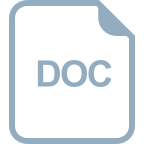
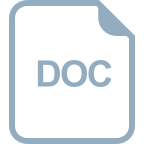
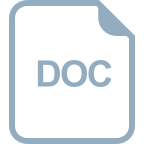
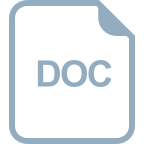
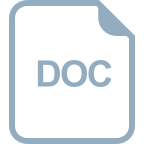

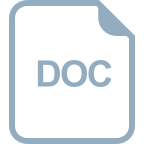
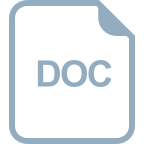
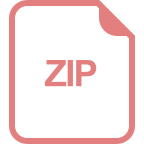
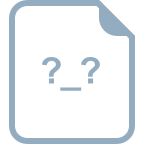
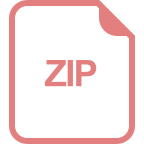