Python请编写程序,模拟某款手游,要求: (1)请自行创建多个"英雄"对象,并初始化每个"英雄"(包括名字,类型,攻击力,生命值等属性)。 (2) 请自行创建“英雄”的战斗技能(例如物理攻击、防御以及魔法伤害) (3) 请自行设计不同的战斗场景,在不同的场景中"英雄"可以攻击另一个"英雄","英雄"的生命值会根据发出攻击的"英雄"的攻击力而下降; (4) "英雄"可以通过吃来增加自身的生命 (5) 英雄"也可以通过打怪来增加自身的生命值; (6)当生命值小于等于0时,表示已被对方杀死。
时间: 2023-07-19 11:27:20 浏览: 83
好的,以下是Python程序,模拟某款手游的代码:
```python
import random
class Hero:
def __init__(self, name, hero_type, attack, hp):
self.name = name
self.hero_type = hero_type
self.attack = attack
self.hp = hp
def attack_hero(self, other_hero):
damage = self.attack
print(f"{self.name}对{other_hero.name}发动攻击,造成了{damage}点伤害!")
other_hero.hp -= damage
if other_hero.hp <= 0:
print(f"{other_hero.name}已被{self.name}杀死!")
def defend(self):
print(f"{self.name}进入防御状态!")
self.hp += 50
def magic_attack(self, other_hero):
damage = self.attack * random.uniform(0.5, 1.5)
print(f"{self.name}释放魔法攻击,对{other_hero.name}造成了{damage}点伤害!")
other_hero.hp -= damage
if other_hero.hp <= 0:
print(f"{other_hero.name}已被{self.name}杀死!")
def eat(self):
hp_gain = random.randint(20, 50)
print(f"{self.name}吃了一口食物,恢复了{hp_gain}点生命值!")
self.hp += hp_gain
def fight_monster(self):
hp_gain = random.randint(50, 100)
print(f"{self.name}击败了一只怪物,获得了{hp_gain}点生命值!")
self.hp += hp_gain
hero1 = Hero("张飞", "战士", 80, 500)
hero2 = Hero("关羽", "战士", 90, 550)
hero3 = Hero("赵云", "法师", 75, 450)
hero4 = Hero("黄忠", "射手", 70, 400)
while True:
print("请选择英雄:")
print("1. 张飞")
print("2. 关羽")
print("3. 赵云")
print("4. 黄忠")
choice = input("请输入序号:")
if choice == "1":
hero = hero1
break
elif choice == "2":
hero = hero2
break
elif choice == "3":
hero = hero3
break
elif choice == "4":
hero = hero4
break
else:
print("输入有误,请重新输入!")
while True:
print("请选择操作:")
print("1. 攻击")
print("2. 防御")
print("3. 魔法攻击")
print("4. 吃")
print("5. 打怪")
choice = input("请输入序号:")
if choice == "1":
while True:
print("请选择攻击对象:")
print("1. 张飞")
print("2. 关羽")
print("3. 赵云")
print("4. 黄忠")
attack_choice = input("请输入序号:")
if attack_choice == "1":
hero.attack_hero(hero1)
break
elif attack_choice == "2":
hero.attack_hero(hero2)
break
elif attack_choice == "3":
hero.attack_hero(hero3)
break
elif attack_choice == "4":
hero.attack_hero(hero4)
break
else:
print("输入有误,请重新输入!")
elif choice == "2":
hero.defend()
elif choice == "3":
while True:
print("请选择攻击对象:")
print("1. 张飞")
print("2. 关羽")
print("3. 赵云")
print("4. 黄忠")
attack_choice = input("请输入序号:")
if attack_choice == "1":
hero.magic_attack(hero1)
break
elif attack_choice == "2":
hero.magic_attack(hero2)
break
elif attack_choice == "3":
hero.magic_attack(hero3)
break
elif attack_choice == "4":
hero.magic_attack(hero4)
break
else:
print("输入有误,请重新输入!")
elif choice == "4":
hero.eat()
elif choice == "5":
hero.fight_monster()
else:
print("输入有误,请重新输入!")
print(f"{hero.name}当前生命值为:{hero.hp}")
if hero.hp <= 0:
print("你已死亡,游戏结束!")
break
```
以上代码实现了一个简单的英雄对战游戏,玩家可以选择不同的英雄进行操作,包括攻击、防御、魔法攻击、吃和打怪等操作,每次操作后英雄的生命值会发生变化,当生命值小于等于0时,表示已被对方杀死,游戏结束。
阅读全文
相关推荐
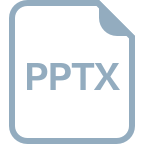
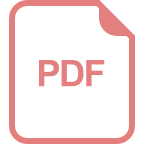
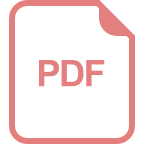
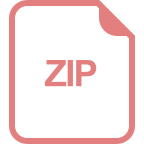
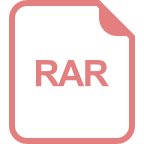
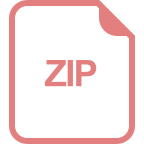
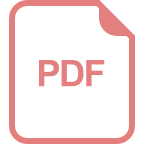
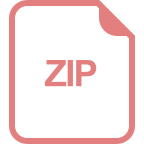
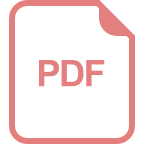
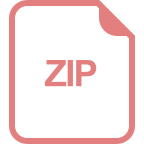
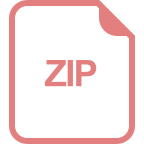
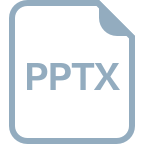
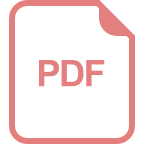
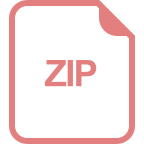
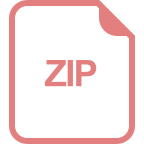
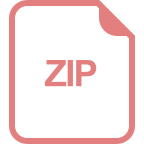
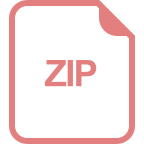
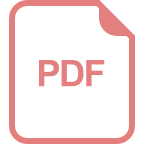
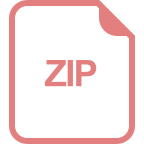