C++代码实现Qtableview 插入一列Qradiobutton
时间: 2023-07-11 19:04:18 浏览: 57
要在QTableView中的特定列中插入QRadioButton,您需要使用QItemDelegate。以下是一个示例代码,其中第2列中的每个单元格都包含一个QRadioButton:
```cpp
#include <QApplication>
#include <QMainWindow>
#include <QStandardItemModel>
#include <QTableView>
#include <QItemDelegate>
#include <QRadioButton>
#include <QHBoxLayout>
class RadioButtonDelegate : public QItemDelegate
{
public:
RadioButtonDelegate(QObject *parent = nullptr) : QItemDelegate(parent) {}
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
if (index.column() == 1) // Only create editor for second column
{
QWidget *editor = new QWidget(parent);
QHBoxLayout *layout = new QHBoxLayout(editor);
layout->setContentsMargins(0, 0, 0, 0);
layout->addWidget(new QRadioButton("Option 1", editor));
editor->setLayout(layout);
return editor;
}
else
{
return QItemDelegate::createEditor(parent, option, index);
}
}
void setEditorData(QWidget *editor, const QModelIndex &index) const override
{
if (index.column() == 1) // Only set data for second column
{
QRadioButton *radioButton = qobject_cast<QRadioButton *>(editor->layout()->itemAt(0)->widget());
radioButton->setChecked(index.data().toBool());
}
else
{
QItemDelegate::setEditorData(editor, index);
}
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override
{
if (index.column() == 1) // Only set data for second column
{
QRadioButton *radioButton = qobject_cast<QRadioButton *>(editor->layout()->itemAt(0)->widget());
model->setData(index, radioButton->isChecked());
}
else
{
QItemDelegate::setModelData(editor, model, index);
}
}
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QStandardItemModel model(4, 2); // 4 rows, 2 columns
model.setHeaderData(0, Qt::Horizontal, "Column 1");
model.setHeaderData(1, Qt::Horizontal, "Column 2");
QTableView tableView;
tableView.setModel(&model);
tableView.setItemDelegate(new RadioButtonDelegate(&tableView));
QMainWindow mainWindow;
mainWindow.setCentralWidget(&tableView);
mainWindow.show();
return a.exec();
}
```
在这个例子中,我们创建了一个名为`RadioButtonDelegate`的自定义QItemDelegate。在`createEditor`函数中,我们创建一个QWidget,将QRadioButton添加到水平布局中,并将其设置为QWidget的布局。然后,我们返回QWidget作为编辑器。在`setEditorData`函数中,我们设置QRadioButton的选中状态,以匹配模型中的数据。在`setModelData`函数中,我们将QRadioButton的选中状态设置为模型中的数据。
在主函数中,我们创建了一个QStandardItemModel,设置了表头,并将其设置为QTableView的模型。我们还将RadioButtonDelegate设置为QTableView的项目委托。
运行程序后,您应该看到一个具有两列和四行的表格,其中第二列包含一个QRadioButton。您可以单击不同的单选按钮,并且模型中的数据应该相应地更新。
相关推荐
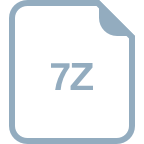
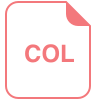













