vue自动加载函数mounted
时间: 2023-08-12 18:09:25 浏览: 59
在Vue中,`mounted`是一个生命周期钩子函数,用于在Vue实例挂载到DOM后执行一些操作。如果你想自动加载函数到`mounted`钩子中,你可以使用 mixin 或者 Vue插件。
1. 使用 mixin:
```javascript
// 定义一个全局的mixin
Vue.mixin({
mounted() {
// 在每个组件的mounted钩子中执行的操作
this.yourFunctionName();
}
});
```
2. 使用Vue插件:
```javascript
// 创建一个Vue插件
const YourPlugin = {
install(Vue) {
Vue.mixin({
mounted() {
// 在每个组件的mounted钩子中执行的操作
this.yourFunctionName();
}
});
}
};
// 在main.js中使用插件
Vue.use(YourPlugin);
```
这样,无论你在哪个组件中使用Vue实例,都会自动调用`yourFunctionName`函数在`mounted`钩子中。当然,你需要将`yourFunctionName`替换为你真正想要执行的函数名。
相关问题
vue页面加载完毕调用函数
在Vue项目中,有两种方案可以在页面加载完毕后立即触发一个函数。
第一种方案是使用created钩子函数。在methods中定义需要触发的函数,然后在created钩子函数中调用这个函数。例如:
```javascript
methods: {
indexs: function() {
this.$http.post('{:url("Index/fun")}')
.then(function(res) {
this.items = res.data;
console.log(res.data);
})
.catch(function(error) {
console.log(error);
});
}
},
created() {
this.indexs(); // 自动加载indexs方法
}
```
在created钩子函数中调用indexs函数,即可在页面渲染完成前触发该函数。
第二种方案是使用mounted钩子函数。在方法中定义需要触发的函数,然后在mounted钩子函数中调用这个函数。例如:
```javascript
methods: {
message: function() {
// 业务逻辑
}
},
mounted: function() {
this.message(); // 需要触发的函数
}
```
在mounted钩子函数中调用message函数,即可在页面渲染完成后触发该函数。
需要注意的是,Vue方法中的箭头函数指向window,所以在实例对象中,箭头函数的父级作用域是window。另外,如果需要在Vue中传递参数给函数,可以使用Vue事件传参的方式进行。
vue3 mounted中加载页面
Vue3的mounted生命周期钩子函数是在组件挂载后调用的函数,也就是在组件头一次渲染完成后执行的函数。
在mounted中加载页面具体可以有以下几种方式:
1. 直接在mounted函数中使用axios等异步请求数据,在请求成功后设置响应数据到data中,从而渲染出页面。
2. 通过调用其他组件/函数,获取数据后再渲染页面,可以把数据交给Vuex进行管理,从而统一管理数据。
3. 通过DOM操作获取数据并渲染页面,但是这种方式需要注意不要和Vue的数据绑定混淆。
在Vue3中,由于使用了Proxy代理机制,mounted函数也有可能出现异步执行的情况,这时可以使用异步方式直接请求数据。另外,由于Vue3不再支持$nextTick方法,因此如果需要在mounted之后获取DOM元素,可以使用setTimeout替代。
相关推荐
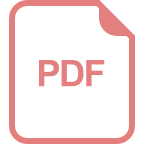
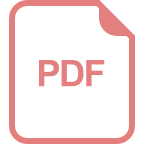
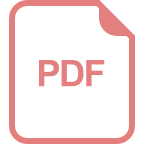
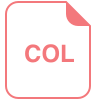











