使用java编写一个程序,对一个字符串中的所有单词进行计数,并按照出现次数从大到小排序输出。 编写一个程序,实现一个简单的计算器,支持加、减、乘、除四种操作。
时间: 2024-03-08 21:46:48 浏览: 59
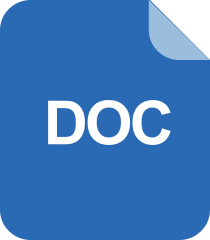
图形用户编程.编写一个简单的计算器程序,要求有菜单,能进行简单的加减乘除运算。

第一个问题的Java代码如下:
```java
import java.util.*;
public class WordCount {
public static void main(String[] args) {
String input = "hello world hello";
Map<String, Integer> wordCount = countWords(input);
List<Map.Entry<String, Integer>> sortedEntries = sortEntries(wordCount);
for (Map.Entry<String, Integer> entry : sortedEntries) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
public static Map<String, Integer> countWords(String input) {
Map<String, Integer> wordCount = new HashMap<>();
String[] words = input.split(" ");
for (String word : words) {
wordCount.put(word, wordCount.getOrDefault(word, 0) + 1);
}
return wordCount;
}
public static List<Map.Entry<String, Integer>> sortEntries(Map<String, Integer> entries) {
List<Map.Entry<String, Integer>> sortedEntries = new ArrayList<>(entries.entrySet());
Collections.sort(sortedEntries, new Comparator<Map.Entry<String, Integer>>() {
@Override
public int compare(Map.Entry<String, Integer> e1, Map.Entry<String, Integer> e2) {
return e2.getValue().compareTo(e1.getValue());
}
});
return sortedEntries;
}
}
```
这个程序中,我们首先定义了一个 `countWords` 方法,它接受一个字符串参数,返回一个 `Map` 对象,记录了每个单词出现的次数。在这个方法中,我们首先使用 `split` 方法将输入字符串按照空格分割成单词数组。然后遍历单词数组,对每个单词使用 `getOrDefault` 方法获取它目前的计数,然后加上1,最后更新到 `wordCount` 中。
接下来,我们定义了一个 `sortEntries` 方法,它接受一个 `Map` 对象,返回一个按照值从大到小排序的 `List` 对象。在这个方法中,我们首先将 `Map` 对象转换为 `List` 对象,然后使用 `Collections.sort` 方法进行排序。排序时,我们使用了一个匿名内部类实现了 `Comparator` 接口,按照值从大到小排序。
最后,在 `main` 方法中,我们演示了如何使用这两个方法进行单词计数并排序输出。
第二个问题的Java代码如下:
```java
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入表达式:");
String expression = scanner.nextLine();
double result = calculate(expression);
System.out.println("计算结果:" + result);
}
public static double calculate(String expression) {
String[] tokens = expression.split(" ");
double operand1 = Double.parseDouble(tokens[0]);
double operand2 = Double.parseDouble(tokens[2]);
String operator = tokens[1];
switch (operator) {
case "+":
return operand1 + operand2;
case "-":
return operand1 - operand2;
case "*":
return operand1 * operand2;
case "/":
return operand1 / operand2;
default:
throw new IllegalArgumentException("不支持的操作符:" + operator);
}
}
}
```
这个程序中,我们首先使用 `Scanner` 类从标准输入读取一个表达式字符串。然后调用 `calculate` 方法计算表达式的结果,并输出到标准输出。
在 `calculate` 方法中,我们首先使用 `split` 方法将表达式按照空格分割成三个部分:第一个操作数、操作符、第二个操作数。然后将两个操作数转换成 `double` 类型,并根据操作符进行相应的计算,最后返回计算结果。注意,如果操作符不是加、减、乘、除中的一个,我们会抛出一个 `IllegalArgumentException` 异常。
阅读全文
相关推荐
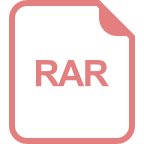


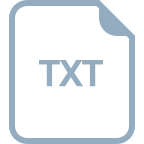
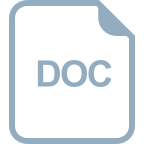
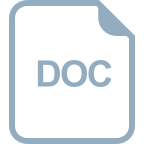
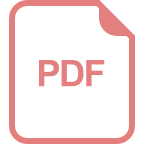
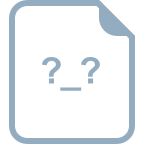
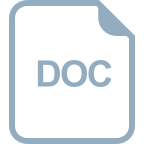
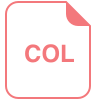
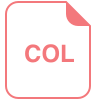
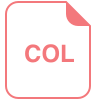
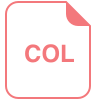



