用Go语言帮我写一个LRU缓存,可以设置过期时间
时间: 2023-03-31 10:00:54 浏览: 203
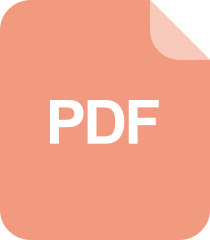
localStorage 设置过期时间的方法实现
好的,我可以帮您写一个Go语言的LRU缓存,以下是示例代码:
```
package main
import (
"container/list"
"fmt"
"time"
)
type CacheItem struct {
key string
value interface{}
expired_at time.Time
}
type LRUCache struct {
capacity int
items map[string]*list.Element
list *list.List
}
func NewLRUCache(capacity int) *LRUCache {
return &LRUCache{
capacity: capacity,
items: make(map[string]*list.Element),
list: list.New(),
}
}
func (c *LRUCache) Get(key string) (interface{}, bool) {
if elem, ok := c.items[key]; ok {
item := elem.Value.(*CacheItem)
if item.expired_at.IsZero() || item.expired_at.After(time.Now()) {
c.list.MoveToFront(elem)
return item.value, true
} else {
c.list.Remove(elem)
delete(c.items, key)
}
}
return nil, false
}
func (c *LRUCache) Set(key string, value interface{}, expired time.Duration) {
if elem, ok := c.items[key]; ok {
c.list.MoveToFront(elem)
elem.Value.(*CacheItem).value = value
elem.Value.(*CacheItem).expired_at = time.Now().Add(expired)
} else {
if len(c.items) >= c.capacity {
tail := c.list.Back()
if tail != nil {
delete(c.items, tail.Value.(*CacheItem).key)
c.list.Remove(tail)
}
}
elem := c.list.PushFront(&CacheItem{key, value, time.Now().Add(expired)})
c.items[key] = elem
}
}
func main() {
cache := NewLRUCache(2)
cache.Set("key1", "value1", time.Second*5)
cache.Set("key2", "value2", time.Second*10)
cache.Set("key3", "value3", time.Second*15)
if value, ok := cache.Get("key1"); ok {
fmt.Println("key1:", value)
} else {
fmt.Println("key1 not found")
}
if value, ok := cache.Get("key2"); ok {
fmt.Println("key2:", value)
} else {
fmt.Println("key2 not found")
}
if value, ok := cache.Get("key3"); ok {
fmt.Println("key3:", value)
} else {
fmt.Println("key3 not found")
}
}
```
这个LRU缓存可以设置过期时间,当缓存中的数据过期时,会自动删除。
阅读全文
相关推荐
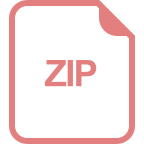
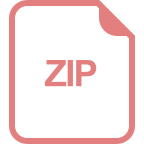
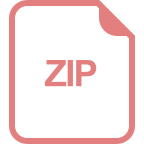
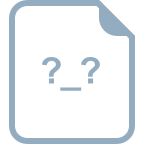
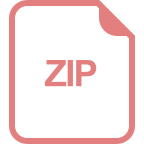
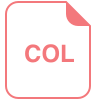
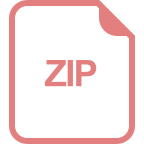
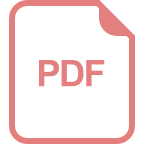
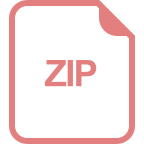
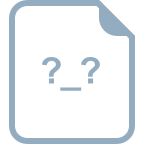
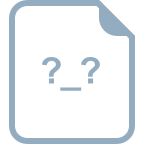
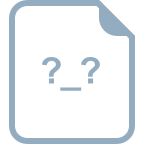
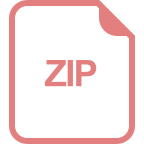
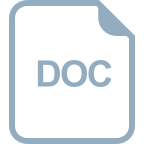
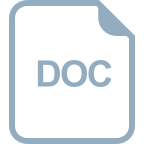
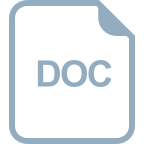
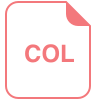