char command[] = "/usr/bin/sn_core.elf getstat"; char line[MAX_LINE_LENGTH]; char *temp_str, *time_str; float temp; char time[MAX_LINE_LENGTH]; int time_ns; char *token; int i = 0; char *array1[50],*array2[50]; FILE *fp = popen(command, "r"); while (fgets(line, MAX_LINE_LENGTH, fp) != NULL) { if ((temp_str = strstr(line, "temp: ")) != NULL) { temp_str += strlen("temp: "); temp = strtof(temp_str, NULL); } // 解析时间 if ((time_str = strstr(line, "Time: ")) != NULL) { time_str += strlen("Time: "); strncpy(time, time_str, MAX_LINE_LENGTH); time[strlen(time) - 1] = '\0'; // 去掉行末的换行符 // 解析纳秒值 token = strtok(time, " +"); while (token != NULL) { if (strchr(token, 'n') != NULL) { time_ns = strtol(token, NULL, 10); break; } token = strtok(NULL, " +"); } } } pclose(fp); // 将解析出的温度和时间存储到数组中 array1[i++] = malloc(sizeof(float)); memcpy(array1[i - 1], &temp, sizeof(float)); array2[i++] = malloc(sizeof(char) * MAX_LINE_LENGTH); strcpy(array2[i - 1], time); // 打印数组内容 for (int j = 0; j < i; j++) { if (j == 0) { printf("温度:%f\n", *((float *)array1[j])); } else { printf("时间:%s\n", array2[j]); } free(array1[j]); free(array2[j]); } 给该代码加一个循环 每一秒循环一次 当第51个值添加到数组时 将数组的第一个值舍弃 以此类推
时间: 2024-02-14 22:10:24 浏览: 52
你可以将代码包装在一个 while 循环中,每次循环等待 1 秒后再进行下一次循环。可以使用一个计数器来记录当前数组中已经存储了多少个值,当计数器达到 50 时,就将数组的第一个值释放掉,并将后面的值全部向前移动一个位置,然后将新的值添加到数组的最后一个位置。以下是修改后的代码:
```
#define MAX_VALUES 50
char command[] = "/usr/bin/sn_core.elf getstat";
char line[MAX_LINE_LENGTH];
char *temp_str, *time_str;
float temp;
char time[MAX_LINE_LENGTH];
int time_ns;
char *token;
int i = 0;
char *array1[MAX_VALUES], *array2[MAX_VALUES];
FILE *fp;
while (1) {
fp = popen(command, "r");
while (fgets(line, MAX_LINE_LENGTH, fp) != NULL) {
if ((temp_str = strstr(line, "temp: ")) != NULL) {
temp_str += strlen("temp: ");
temp = strtof(temp_str, NULL);
}
if ((time_str = strstr(line, "Time: ")) != NULL) {
time_str += strlen("Time: ");
strncpy(time, time_str, MAX_LINE_LENGTH);
time[strlen(time) - 1] = '\0';
token = strtok(time, " +");
while (token != NULL) {
if (strchr(token, 'n') != NULL) {
time_ns = strtol(token, NULL, 10);
break;
}
token = strtok(NULL, " +");
}
}
}
pclose(fp);
// 将解析出的温度和时间存储到数组中
if (i < MAX_VALUES) {
array1[i] = malloc(sizeof(float));
memcpy(array1[i], &temp, sizeof(float));
array2[i] = malloc(sizeof(char) * MAX_LINE_LENGTH);
strcpy(array2[i], time);
i++;
} else {
// 释放第一个值
free(array1[0]);
free(array2[0]);
// 将剩余的值向前移动一个位置
memmove(array1, array1 + 1, (MAX_VALUES - 1) * sizeof(char *));
memmove(array2, array2 + 1, (MAX_VALUES - 1) * sizeof(char *));
// 添加新的值到最后一个位置
array1[MAX_VALUES - 1] = malloc(sizeof(float));
memcpy(array1[MAX_VALUES - 1], &temp, sizeof(float));
array2[MAX_VALUES - 1] = malloc(sizeof(char) * MAX_LINE_LENGTH);
strcpy(array2[MAX_VALUES - 1], time);
}
// 打印数组内容
for (int j = 0; j < i; j++) {
if (j == 0) {
printf("温度:%f\n", *((float *)array1[j]));
} else {
printf("时间:%s\n", array2[j]);
}
}
sleep(1);
}
```
阅读全文
相关推荐
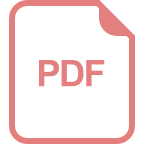
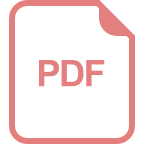
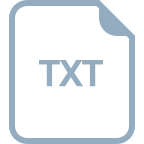








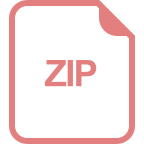
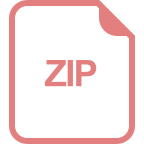