这个例子中,举例说明一下其他特定于用户类型的属性和操作
时间: 2024-04-15 11:30:49 浏览: 15
当使用类模板设计模式创建管理员用户和访客用户时,可以为每个特定用户类型添加特定的属性和操作。以下是一个示例,展示了如何为管理员用户和访客用户添加特定的属性和操作:
```cpp
#include <iostream>
#include <string>
// 用户类模板
template <typename UserType>
class User {
private:
std::string username;
std::string password;
public:
User(const std::string& username, const std::string& password)
: username(username), password(password) {}
void login() {
std::cout << "User Type: " << UserType::userType << std::endl;
std::cout << "Username: " << username << std::endl;
std::cout << "Password: " << password << std::endl;
// 具体的登录逻辑
UserType::specificOperation(); // 调用特定用户类型的操作
}
};
// 管理员用户类
struct AdminUser {
static constexpr const char* userType = "Admin";
static void specificOperation() {
std::cout << "Performing admin-specific operation." << std::endl;
// 具体的管理员用户操作
}
// 其他管理员用户的特有属性和操作
};
// 访客用户类
struct GuestUser {
static constexpr const char* userType = "Guest";
static void specificOperation() {
std::cout << "Performing guest-specific operation." << std::endl;
// 具体的访客用户操作
}
// 其他访客用户的特有属性和操作
};
int main() {
User<AdminUser> admin("admin", "admin123");
admin.login();
User<GuestUser> guest("guest", "guest123");
guest.login();
return 0;
}
```
在上面的示例中,我们在`AdminUser`和`GuestUser`结构体中添加了一个名为`specificOperation`的静态成员函数,用于执行特定用户类型的操作。在`User`类模板的`login`方法中,我们通过调用`UserType::specificOperation()`来执行特定用户类型的操作。
这样,每个用户类型都可以有自己特有的属性和操作。例如,管理员用户可以在`specificOperation`方法中执行管理员特有的操作,而访客用户可以在自己的`specificOperation`方法中执行访客特有的操作。通过模板设计模式,我们可以方便地创建不同类型的用户对象,并在登录时执行特定用户类型的操作。
相关推荐
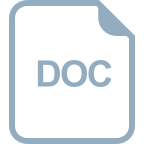
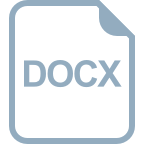
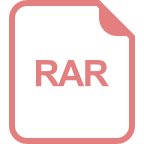












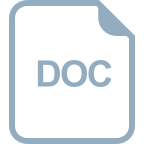
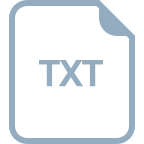
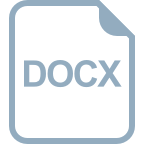