Basedao封装增、删、查、改作方法
时间: 2023-08-14 12:04:41 浏览: 283
好的,下面是一个简单的BaseDao类,封装了增加、删除、查询、更新四个方法:
```
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class BaseDao {
// 定义数据库连接对象
private Connection conn = null;
// 构造方法,初始化数据库连接对象
public BaseDao() {
conn = DBUtil.getConnection();
}
// 增加数据方法
public int add(String sql, Object... params) {
int count = 0;
PreparedStatement pstmt = null;
try {
pstmt = conn.prepareStatement(sql);
// 设置占位符参数
for (int i = 0; i < params.length; i++) {
pstmt.setObject(i + 1, params[i]);
}
// 执行增加操作
count = pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(null, pstmt, null);
}
return count;
}
// 删除数据方法
public int delete(String sql, Object... params) {
int count = 0;
PreparedStatement pstmt = null;
try {
pstmt = conn.prepareStatement(sql);
// 设置占位符参数
for (int i = 0; i < params.length; i++) {
pstmt.setObject(i + 1, params[i]);
}
// 执行删除操作
count = pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(null, pstmt, null);
}
return count;
}
// 查询数据方法
public ResultSet query(String sql, Object... params) {
ResultSet rs = null;
PreparedStatement pstmt = null;
try {
pstmt = conn.prepareStatement(sql);
// 设置占位符参数
for (int i = 0; i < params.length; i++) {
pstmt.setObject(i + 1, params[i]);
}
// 执行查询操作
rs = pstmt.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
return rs;
}
// 更新数据方法
public int update(String sql, Object... params) {
int count = 0;
PreparedStatement pstmt = null;
try {
pstmt = conn.prepareStatement(sql);
// 设置占位符参数
for (int i = 0; i < params.length; i++) {
pstmt.setObject(i + 1, params[i]);
}
// 执行更新操作
count = pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(null, pstmt, null);
}
return count;
}
}
```
在使用该类时,可以通过继承的方式来实现具体的操作,例如:
```
public class UserDao extends BaseDao {
// 添加用户
public int addUser(User user) {
String sql = "insert into user(username, password, age) values(?,?,?)";
return super.add(sql, user.getUsername(), user.getPassword(), user.getAge());
}
// 删除用户
public int deleteUser(int id) {
String sql = "delete from user where id=?";
return super.delete(sql, id);
}
// 查询用户
public User getUser(int id) {
User user = null;
String sql = "select * from user where id=?";
ResultSet rs = super.query(sql, id);
try {
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setAge(rs.getInt("age"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return user;
}
// 更新用户
public int updateUser(User user) {
String sql = "update user set username=?, password=?, age=? where id=?";
return super.update(sql, user.getUsername(), user.getPassword(), user.getAge(), user.getId());
}
}
```
这样,我们就可以通过继承BaseDao类来实现具体的增、删、查、改操作了。
阅读全文
相关推荐

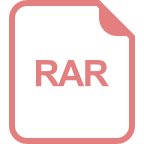













