使用这些javabean serlvet jsp dao完成图书增加的功能
时间: 2024-05-07 10:22:38 浏览: 55
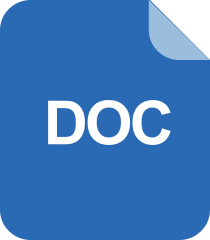
基于JSP技术的图书馆管理系统
好的,下面是一个简单的示例:
1. 首先,创建一个Book类作为JavaBean,包含图书的属性:
```java
public class Book {
private String title;
private String author;
private String publisher;
private int price;
// getter和setter方法
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}
```
2. 创建一个BookDao类,用于处理数据库中的图书数据:
```java
public class BookDao {
// 数据库连接
private Connection conn;
// 构造函数
public BookDao() {
try {
// 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 获取数据库连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/bookstore", "root", "password");
} catch (Exception e) {
e.printStackTrace();
}
}
// 添加图书
public void addBook(Book book) {
try {
// 创建PreparedStatement对象
PreparedStatement ps = conn.prepareStatement("INSERT INTO books (title, author, publisher, price) VALUES (?, ?, ?, ?)");
// 设置参数
ps.setString(1, book.getTitle());
ps.setString(2, book.getAuthor());
ps.setString(3, book.getPublisher());
ps.setInt(4, book.getPrice());
// 执行SQL语句
ps.executeUpdate();
// 关闭PreparedStatement对象
ps.close();
} catch (Exception e) {
e.printStackTrace();
}
}
// 关闭数据库连接
public void close() {
try {
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
3. 创建一个AddBookServlet类,用于处理用户提交的表单数据:
```java
public class AddBookServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取表单数据
String title = request.getParameter("title");
String author = request.getParameter("author");
String publisher = request.getParameter("publisher");
int price = Integer.parseInt(request.getParameter("price"));
// 创建Book对象
Book book = new Book();
book.setTitle(title);
book.setAuthor(author);
book.setPublisher(publisher);
book.setPrice(price);
// 创建BookDao对象
BookDao dao = new BookDao();
// 添加图书
dao.addBook(book);
// 关闭数据库连接
dao.close();
// 跳转到成功页面
response.sendRedirect("success.jsp");
}
}
```
4. 创建一个add_book.jsp页面,用于显示添加图书的表单:
```html
<html>
<head>
<title>Add Book</title>
</head>
<body>
<h1>Add Book</h1>
<form action="AddBookServlet" method="post">
<table>
<tr>
<td>Title:</td>
<td><input type="text" name="title"></td>
</tr>
<tr>
<td>Author:</td>
<td><input type="text" name="author"></td>
</tr>
<tr>
<td>Publisher:</td>
<td><input type="text" name="publisher"></td>
</tr>
<tr>
<td>Price:</td>
<td><input type="text" name="price"></td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="Add"></td>
</tr>
</table>
</form>
</body>
</html>
```
5. 创建一个success.jsp页面,用于显示添加图书成功的消息:
```html
<html>
<head>
<title>Success</title>
</head>
<body>
<h1>Success</h1>
<p>The book has been added successfully!</p>
</body>
</html>
```
这样,当用户访问add_book.jsp页面并提交表单后,将会调用AddBookServlet类的doPost方法,将图书数据保存到数据库中,然后跳转到success.jsp页面显示成功消息。
阅读全文
相关推荐
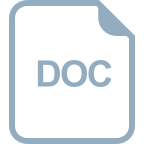
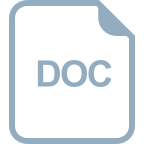
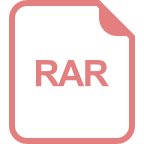
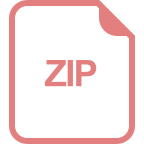
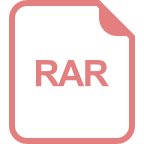
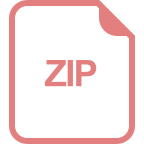
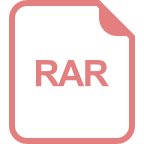
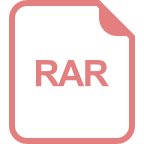
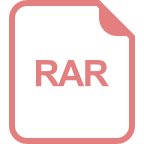
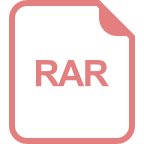
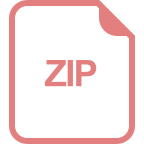

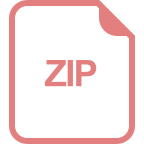
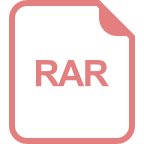
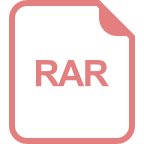
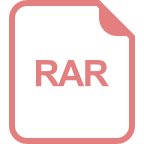
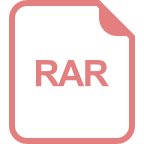