C# 利用接口(Interface) 通过tcp协议与机器人通信
时间: 2024-10-26 10:16:52 浏览: 54
在C#中,利用接口(Interface)通过TCP协议与机器人通信,可以实现一种松耦合的设计模式,让代码更具灵活性。以下是基本步骤:
1. **定义接口**:首先,创建一个表示机器人通信接口的接口,包含发送消息(SendCommand)、接收响应(ReceiveResponse)等抽象方法。例如:
```csharp
public interface IRobotCommunication
{
void SendCommand(string command);
string ReceiveResponse();
}
```
2. **实现接口**:对于每个需要连接机器人的客户端,比如控制台应用或者服务端程序,都需要实现这个接口,提供具体的TCP通信实现:
- 客户端(Client):
```csharp
public class TcpClientAdapter : IRobotCommunication
{
private readonly TcpClient tcpClient;
public TcpClientAdapter(TcpClient tcpClient)
{
this.tcpClient = tcpClient;
}
public void SendCommand(string command) => SendData(Encoding.UTF8.GetBytes(command));
public string ReceiveResponse() => Encoding.UTF8.GetString(ReceiveData());
// 实现数据传输的具体方法,如SendData和ReceiveData
}
```
- 服务器(Server):
```csharp
public class TcpServerHandler : IRobotCommunication
{
private readonly TcpListener listener;
public TcpServerHandler(int port)
{
listener = new TcpListener(IPAddress.Any, port);
listener.Start();
}
public void SendCommand(string command) => HandleTcpConnection(client => client.Send(Encoding.UTF8.GetBytes(command)));
public string ReceiveResponse() => ReadFromTcpConnection().ToString();
// 实现处理连接、读取数据的具体方法,如HandleTcpConnection和ReadFromTcpConnection
}
```
3. **使用接口**:在你的主程序中,不需要关心底层通信细节,只需持有`IRobotCommunication`类型的引用并与之交互:
```csharp
IRobotCommunication robot = new TcpClientAdapter(tcpClient); // 对于客户端
// 或者
IRobotCommunication robot = new TcpServerHandler(port); // 对于服务器
robot.SendCommand("Hello Robot");
string response = robot.ReceiveResponse();
```
阅读全文
相关推荐
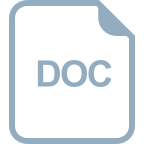
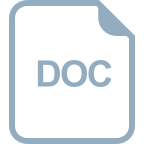
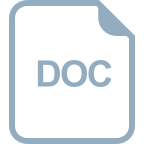
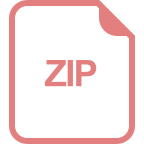


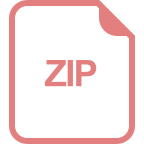
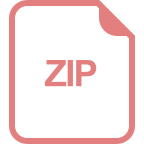
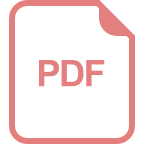
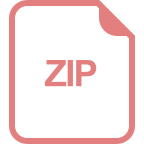
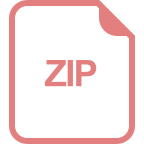
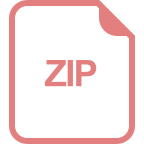
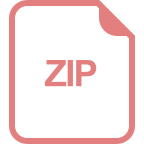
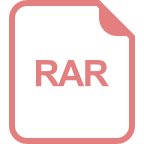
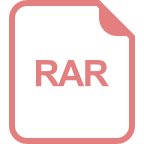
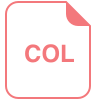
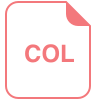
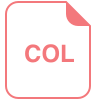
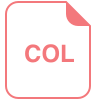