c# 判断文件夹是否为空
时间: 2023-08-02 08:38:09 浏览: 87
可以使用以下代码来判断文件夹是否为空:
```csharp
string path = @"C:\folder\subfolder";
if (Directory.GetFiles(path).Length == 0 && Directory.GetDirectories(path).Length == 0)
{
Console.WriteLine("The folder is empty.");
}
else
{
Console.WriteLine("The folder is not empty.");
}
```
其中,`Directory.GetFiles(path)` 返回指定路径下的文件列表,`Directory.GetDirectories(path)` 返回指定路径下的文件夹列表。如果这两个列表的长度都为0,那么就可以判断该文件夹为空。
相关问题
使用C#判断文件夹是否为空
你可以使用C#中的Directory类提供的静态方法GetFileSystemEntries()方法来获取目录中的文件和文件夹列表,然后判断该列表是否为空。以下是一个示例代码:
```csharp
using System.IO;
// 检查文件夹是否为空
public bool IsDirectoryEmpty(string path)
{
string[] files = Directory.GetFileSystemEntries(path);
return (files.Length == 0);
}
```
在上面的示例代码中,IsDirectoryEmpty()方法接收一个字符串参数作为目录路径,然后使用Directory类的GetFileSystemEntries()方法获取该目录中的所有文件和文件夹。最后,该方法返回一个布尔值,指示该目录是否为空。
C#判断文件夹是否存在
在C#中,可以使用`Directory`类来判断文件夹是否存在。具体来说,可以使用`Directory.Exists()`方法来判断文件夹是否存在,该方法接受文件夹路径作为参数,返回一个布尔值,表示该文件夹是否存在。
以下是一个示例代码:
```csharp
using System.IO;
// 判断文件夹是否存在
string folderPath = "C:\\Users\\User\\Documents\\MyFolder";
if (Directory.Exists(folderPath))
{
Console.WriteLine("文件夹已存在");
}
else
{
Console.WriteLine("文件夹不存在");
}
```
在上面的代码中,我们使用`Directory.Exists()`方法来判断指定路径的文件夹是否存在,如果存在则输出“文件夹已存在”,否则输出“文件夹不存在”。
相关推荐
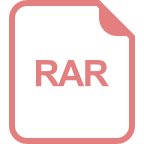












