Java 代码获取 运营在列表中下载视频的进度 代码
时间: 2024-04-21 22:26:59 浏览: 16
以下是一个简单的Java代码示例,用于获取运营在列表中下载视频的进度:
```
import java.io.File;
import java.io.InputStream;
import java.io.RandomAccessFile;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class VideoDownloader {
private static final int THREAD_POOL_SIZE = 5; // 线程池大小
private static final int BUFFER_SIZE = 1024 * 1024; // 缓冲区大小
private static final String SAVE_PATH = "D:/videos/"; // 视频保存路径
private List<Video> videoList; // 视频列表
private ExecutorService threadPool; // 线程池
private int totalSize; // 视频总大小
private int downloadedSize; // 已下载的视频大小
public VideoDownloader(List<Video> videoList) {
this.videoList = videoList;
threadPool = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
}
public void start() {
// 计算视频总大小
totalSize = 0;
for (Video video : videoList) {
totalSize += video.getSize();
}
// 创建本地保存目录
File saveDir = new File(SAVE_PATH);
if (!saveDir.exists()) {
saveDir.mkdirs();
}
// 下载视频
downloadedSize = 0;
for (Video video : videoList) {
threadPool.execute(new DownloadTask(video));
}
}
public synchronized int getProgress() {
return (int) (downloadedSize * 100.0 / totalSize);
}
private class DownloadTask implements Runnable {
private Video video;
public DownloadTask(Video video) {
this.video = video;
}
@Override
public void run() {
try {
URL url = new URL(video.getUrl());
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5000);
// 设置下载区间
int startPos = video.getStartPos();
int endPos = video.getEndPos();
conn.setRequestProperty("Range", "bytes=" + startPos + "-" + endPos);
// 获取视频输入流
InputStream is = conn.getInputStream();
// 创建本地文件
File file = new File(SAVE_PATH + video.getName());
RandomAccessFile raf = new RandomAccessFile(file, "rwd");
// 设置文件写入位置
raf.seek(startPos);
// 缓冲区
byte[] buffer = new byte[BUFFER_SIZE];
int len;
// 下载视频数据
while ((len = is.read(buffer)) != -1) {
raf.write(buffer, 0, len);
downloadedSize += len;
}
// 关闭流
is.close();
raf.close();
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
// 创建视频列表
List<Video> videoList = new ArrayList<>();
videoList.add(new Video("video1.mp4", "http://example.com/video1.mp4", 0, 102400));
videoList.add(new Video("video2.mp4", "http://example.com/video2.mp4", 102401, 204800));
videoList.add(new Video("video3.mp4", "http://example.com/video3.mp4", 204801, 307200));
videoList.add(new Video("video4.mp4", "http://example.com/video4.mp4", 307201, 409600));
videoList.add(new Video("video5.mp4", "http://example.com/video5.mp4", 409601, 512000));
// 开始下载视频
VideoDownloader downloader = new VideoDownloader(videoList);
downloader.start();
// 显示下载进度
while (downloader.getProgress() < 100) {
System.out.printf("Download progress: %d%%\n", downloader.getProgress());
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Download complete!");
}
}
class Video {
private String name;
private String url;
private int startPos;
private int endPos;
public Video(String name, String url, int startPos, int endPos) {
this.name = name;
this.url = url;
this.startPos = startPos;
this.endPos = endPos;
}
public String getName() {
return name;
}
public String getUrl() {
return url;
}
public int getStartPos() {
return startPos;
}
public int getEndPos() {
return endPos;
}
public int getSize() {
return endPos - startPos + 1;
}
}
```
在上面的代码中,我们使用了Java中的多线程技术来实现视频的并行下载。在主线程中创建了一个线程池,用于管理下载视频的线程。对于每个视频,我们创建了一个下载线程,并将其加入线程池中。在下载线程中,我们使用Java中的URLConnection类打开视频的URL连接,获取视频的大小,并使用Java中的RandomAccessFile类创建一个本地文件,用于存储下载的视频数据。最后,我们在主线程中定时获取线程池中每个下载线程的下载进度,并计算出整个列表的下载进度,并将下载进度显示给用户。
相关推荐
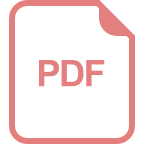
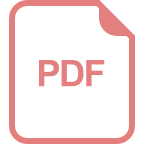














