怎么用传入的字典值自动写入request.POST?
时间: 2023-08-22 22:08:36 浏览: 44
可以使用Python的`dict`类型的`update()`方法将一个字典的键值对更新/合并到另一个字典中,从而将传入的字典值自动写入`request.POST`中。
示例代码如下:
```python
def my_view(request):
if request.method == 'POST':
# 将传入的字典值自动写入request.POST
request.POST.update(request.GET)
# 在此处进行POST请求处理
# ...
```
在上面的示例代码中,我们假设传入的字典值在`request.GET`中,可以使用`update()`方法将`request.GET`中的键值对更新到`request.POST`中,从而自动写入传入的字典值。
相关问题
public string post_urlencoded(string url, string cookies, string postData) { HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url); try { request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded;"; if (cookies != "") { request.Headers.Add("Cookie", cookies); } // 参数 StreamWriter writer = new StreamWriter(request.GetRequestStream(), Encoding.GetEncoding("EUC-KR")); writer.Write(postData); writer.Close(); HttpWebResponse response = (HttpWebResponse)request.GetResponse(); string encoding = response.ContentEncoding; if (encoding == null || encoding.Length < 1) { encoding = "EUC-KR"; //默认编码 } StreamReader reader = new StreamReader(response.GetResponseStream(), Encoding.GetEncoding(encoding)); string retString = reader.ReadToEnd(); reader.Close(); reader.Dispose(); response.Close(); response.Dispose(); return retString; } catch (WebException ex) { HttpWebResponse response = (HttpWebResponse)ex.Response; StreamReader reader = new StreamReader(response.GetResponseStream(), Encoding.GetEncoding("euc-kr")); string retString = reader.ReadToEnd(); return "error"; } finally { if (request != null) { request.Abort(); } } }什么意思
这是一个基于.NET Framework的C#代码片段,用于发送POST请求并获取响应数据。该方法接收三个参数:
- `url`:请求的URL地址。
- `cookies`:请求需要使用的Cookie,如果没有则传空字符串。
- `postData`:请求参数,格式为"x-www-form-urlencoded"。
该方法使用`HttpWebRequest`类来创建POST请求,并在请求头中设置`Content-Type`为`application/x-www-form-urlencoded`来表示请求参数的格式。如果有传入Cookie,则在请求头中添加Cookie信息。然后使用`StreamWriter`类向请求流中写入POST数据,POST数据使用EUC-KR编码。接着发送请求,获取响应数据,判断响应数据的编码格式并使用相应的编码格式解码响应数据。最后返回解码后的响应数据。
如果发生WebException异常,则代表请求失败,此时从异常响应中获取响应数据。如果请求成功,则返回解码后的响应数据,否则返回"error"。
HttpPost 数据输入流写入参数
### 回答1:
可以使用 Java 中的 Apache HttpClient 库来实现 HttpPost 请求,然后使用 setEntity 方法设置请求体,将参数写入数据输入流中。以下是示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.InputStreamEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class HttpPostExample {
public static void main(String[] args) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://example.com/api");
// 设置请求体
String requestBody = "param1=value1¶m2=value2";
InputStream inputStream = new ByteArrayInputStream(requestBody.getBytes(StandardCharsets.UTF_8));
HttpEntity httpEntity = new InputStreamEntity(inputStream);
httpPost.setEntity(httpEntity);
CloseableHttpResponse response = httpClient.execute(httpPost);
try {
HttpEntity entity = response.getEntity();
if (entity != null) {
String responseString = EntityUtils.toString(entity);
System.out.println(responseString);
}
} finally {
response.close();
}
}
}
```
在上面的示例中,首先创建了一个 CloseableHttpClient 对象,然后创建 HttpPost 对象并指定请求的 URL。接着将要发送的参数字符串转换为字节数组并通过 ByteArrayInputStream 将其转换为 InputStream 对象。最后创建 InputStreamEntity 对象,并使用 HttpPost 的 setEntity 方法将其设置为请求体。最后执行请求并读取响应。
### 回答2:
HttpPost是一个用于发送HTTP POST请求的类。当需要将数据写入参数时,可以借助HttpPost的数据输入流进行操作。
一般步骤如下:
1. 创建HttpPost对象,并设置请求的URL地址。
2. 创建一个NameValuePair列表,用于存放待写入的参数。
3. 将待写入的参数以键值对的形式添加到NameValuePair列表中。
4. 通过HttpPost对象获取请求的实体,并设置实体的数据为NameValuePair列表的内容。
5. 发送请求,并获取响应结果。
具体实现的代码如下:
```java
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
public class HttpPostExample {
public static void main(String[] args) {
String url = "http://example.com/api/endpoint";
HttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
// 创建参数列表
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("param1", "value1"));
params.add(new BasicNameValuePair("param2", "value2"));
// 设置实体的数据为参数列表的内容
httpPost.setEntity(new UrlEncodedFormEntity(params, "UTF-8"));
// 发送请求并获取响应
HttpResponse response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
// 处理响应结果
if (entity != null) {
InputStream inputStream = entity.getContent();
String result = EntityUtils.toString(entity);
// 处理返回的结果
System.out.println(result);
inputStream.close();
}
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
httpClient.getConnectionManager().shutdown();
}
}
}
```
以上代码中,创建了一个HttpPost对象,设置了请求的URL地址,创建了一个NameValuePair列表,将待写入的参数添加到列表中。然后通过setEntity方法将参数列表作为实体的数据进行设置。最后,发送请求并获取响应结果,将其转换为字符串并进行处理。
### 回答3:
通过HttpPost请求发送数据时,可以使用输入流将参数写入请求体中。
首先,需要创建一个HttpPost对象,设置请求URL。
然后,创建一个DefaultHttpClient对象,并将HttpPost对象传入。
接下来,创建一个List<NameValuePair>集合,用于存储请求参数。
然后,将参数按照键值对的方式添加到集合中,例如:
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("username", "张三"));
params.add(new BasicNameValuePair("password", "123456"));
接着,通过UrlEncodedFormEntity将参数集合编码成URL格式,并设置给HttpPost对象。
最后,执行请求并获取响应结果。
下面是完整的示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
public class HttpPostExample {
public static void main(String[] args) {
String url = "http://example.com/api/login";
// 创建HttpPost对象
HttpPost httpPost = new HttpPost(url);
// 创建DefaultHttpClient对象
DefaultHttpClient httpClient = new DefaultHttpClient();
// 设置请求参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("username", "张三"));
params.add(new BasicNameValuePair("password", "123456"));
try {
// 将参数编码并设置给HttpPost对象
httpPost.setEntity(new UrlEncodedFormEntity(params, "UTF-8"));
// 执行请求
HttpResponse response = httpClient.execute(httpPost);
// 获取响应结果
HttpEntity entity = response.getEntity();
if (entity != null) {
InputStream inputStream = entity.getContent();
String result = EntityUtils.toString(entity, "UTF-8");
System.out.println(result);
// 关闭输入流
inputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
// 关闭DefaultHttpClient对象
httpClient.close();
}
}
```
这是一个简单的HttpPost请求示例,使用输入流将参数写入请求体中,并执行请求获取响应结果。
相关推荐
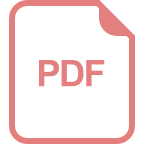
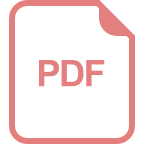
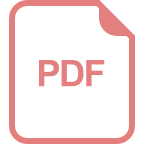












