Android如何将数据库中的byte数组转成图片显示在activity上
时间: 2024-05-26 19:10:20 浏览: 11
你可以通过将byte数组转成Bitmap对象,再将其显示在ImageView上实现这个功能。具体实现步骤如下:
1. 从数据库中获取byte数组数据。
2. 使用BitmapFactory.decodeByteArray() 方法将byte数组转成Bitmap对象。
3. 将Bitmap对象设置到ImageView上,通过setImageBitmap() 方法实现。
代码示例:
byte[] data = // 从数据库中获取byte数组数据
Bitmap bitmap = BitmapFactory.decodeByteArray(data, 0, data.length);
ImageView imageView = findViewById(R.id.image_view);
imageView.setImageBitmap(bitmap);
希望这个回答可以帮到你。
相关问题
byte数组转换成图片
### 回答1:
将byte数组转换为图片需要使用IO流进行读写操作。可以使用Java的ByteArrayInputStream类将byte数组读入到输入流中,然后使用ImageIO类的read方法读取图像文件,最后使用ImageIO类的write方法将读取到的图像文件写入到输出流中。
示例代码如下:
```
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
public class ByteArrayToImage {
public static void main(String[] args) {
byte[] byteArray = {1,2,3,4,5,6,7,8,9};
BufferedImage image = null;
try {
ByteArrayInputStream bis = new ByteArrayInputStream(byteArray);
image = ImageIO.read(bis);
ImageIO.write(image, "jpg", new File("output.jpg"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上面的程序将byte数组转换成图片文件并写入到本地的一个叫做output.jpg的文件中.
其中, byteArray为要转换的byte数组,后面的“jpg”是图片格式, "output.jpg" 是输出文件的路径。
### 回答2:
byte数组转换成图片,可以通过以下步骤实现:
1. 首先,我们需要创建一个空的BufferedImage对象,用于存储图片的像素数据。
2. 然后,可以使用ImageIO类的read方法,将byte数组转换成BufferedImage对象。该方法需要传入一个ByteArrayInputStream对象作为参数,将byte数组包装成输入流。
3. 接下来,我们可以根据BufferedImage对象的getWidth和getHeight方法,获取图片的宽度和高度。
4. 创建一个Graphics对象,通过调用BufferedImage对象的createGraphics方法得到。这个Graphics对象可以用于后续图片的绘制操作。
5. 调用Graphics对象的drawImage方法,将BufferedImage对象绘制到Graphics对象上。
6. 最后,可以通过ImageIO类的write方法,将BufferedImage对象保存为图片文件。该方法需要传入一个File对象和一个格式字符串作为参数。
7. 如果想要以其他格式保存图片,可以在写入之前使用ImageIO类的setUseCache方法设置为false,并传入其他格式的Writer对象。
通过以上步骤,我们就可以将byte数组转换成图片并保存到文件中。
### 回答3:
将byte数组转换为图片需要经过以下几个步骤:
1. 首先,需要获取到byte数组作为输入。这个byte数组通常是从文件、数据库或网络中读取出来的图片数据,它是以字节序列的形式保存的。
2. 接下来,我们需要创建一个BufferedImage对象。BufferedImage是Java中处理图片的类,它可以用来生成、修改或合成图片。
3. 然后,我们可以使用ImageIO类的静态方法read来读取byte数组并将其转换为BufferedImage对象。这个方法接受一个输入流作为参数,由于我们的输入是一个byte数组,所以我们可以使用ByteArrayInputStream类将byte数组转换为输入流。
4. 接下来,我们可以根据BufferedImage对象的宽度和高度创建一个Graphics2D对象,并使用其drawImage方法绘制图片。在这一步中,我们可以设置图像的大小、颜色、透明度等,以便于我们对图片进行进一步的处理。
5. 最后,我们可以使用ImageIO类的静态方法write将BufferedImage对象以指定的格式写入到一个输出流中。这个输出流可以是一个文件输出流,以将图片保存到本地文件中;也可以是一个字节数组输出流,以便于我们将图片数据传输到网络或其他地方。
通过以上步骤,我们可以将byte数组转换成图片,并根据需求对图片进行进一步的处理和操作。在实际应用中,我们可以根据具体的需求选择合适的图片格式和处理方法,以达到最佳的效果和性能。
C#把byte数组转换成图片
可以使用C#中的System.Drawing.Bitmap类来将byte数组转换为图片。具体实现步骤如下:
1. 将byte数组转换为内存流(MemoryStream)对象:
```
byte[] bytes = ...
MemoryStream ms = new MemoryStream(bytes);
```
2. 使用Bitmap类从内存流中创建图片对象:
```
Bitmap bitmap = new Bitmap(ms);
```
3. 可以将图片对象保存到本地文件或显示在窗口中:
```
bitmap.Save("image.jpg", ImageFormat.Jpeg); // 保存到本地文件
pictureBox1.Image = bitmap; // 显示在PictureBox控件中
```
注意:在使用完内存流对象后,应该调用Dispose()方法释放资源:
```
ms.Dispose();
```
相关推荐
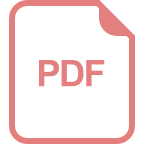
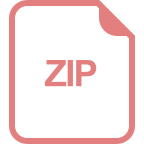
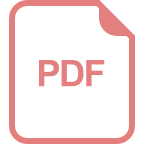












