用c++数据结构O(n+m) 的做法来解决这个问题,不能使用stl容器,给出C++代码:给一个n (1≤n≤106) 个点m(1≤m≤106) 条边的无向图,求 s 到 t 的最短路的长度。 数据保证,s 出发可以到达 t。时间限制: 1000 ms 内存空间限制: 128 MiB
时间: 2024-02-16 18:00:08 浏览: 120
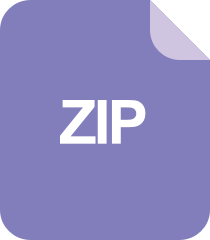
最短路径的C++算法
可以使用 Dijkstra 算法来求解最短路的长度,时间复杂度为 O(nlogn+m)。
具体实现如下:
```c++
#include <iostream>
#include <cstring>
using namespace std;
const int N = 1e6 + 10;
const int M = 2e6 + 10;
const int INF = 0x3f3f3f3f;
int h[N], e[M], w[M], ne[M], idx;
int dist[N];
bool st[N];
int n, m;
void add(int a, int b, int c)
{
e[idx] = b, w[idx] = c, ne[idx] = h[a], h[a] = idx ++;
}
void dijkstra(int start)
{
memset(dist, 0x3f, sizeof dist);
memset(st, false, sizeof st);
dist[start] = 0;
for (int i = 0; i < n; i ++)
{
int t = -1;
for (int j = 1; j <= n; j ++)
if (!st[j] && (t == -1 || dist[t] > dist[j]))
t = j;
st[t] = true;
for (int j = h[t]; j != -1; j = ne[j])
{
int k = e[j];
if (dist[k] > dist[t] + w[j])
dist[k] = dist[t] + w[j];
}
}
}
int main()
{
cin >> n >> m;
memset(h, -1, sizeof h);
while (m --)
{
int a, b, c;
cin >> a >> b >> c;
add(a, b, c);
add(b, a, c);
}
int s, t;
cin >> s >> t;
dijkstra(s);
cout << dist[t] << endl;
return 0;
}
```
阅读全文
相关推荐
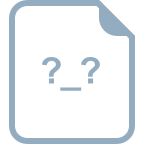
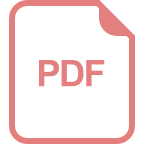
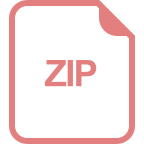
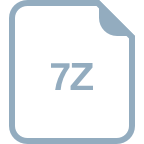
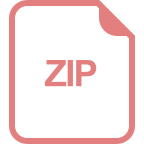
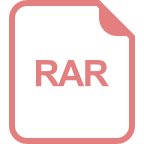
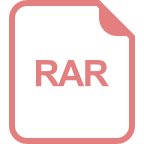
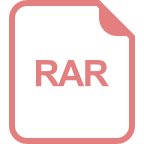
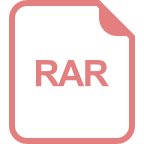
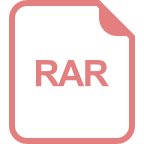
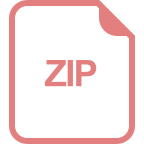
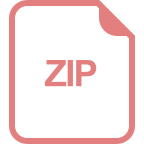
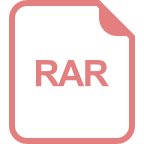
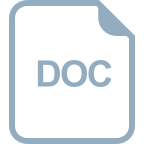
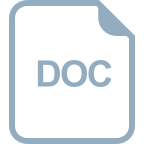
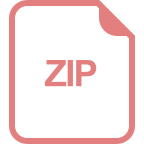
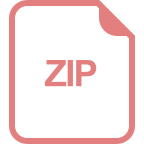