Java Swing 实现购物小程序的代码
时间: 2023-11-11 09:04:17 浏览: 112
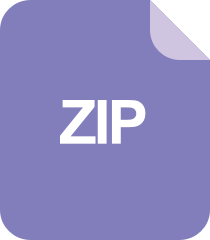
基于JavaSwing超市购物进销存系统(源代码+数据库+数据库表字段说明)
以下是一个简单的购物小程序的 Java Swing 代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ShoppingApp extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JLabel title, item1, item2, item3, item4, totalPrice;
private JTextField qty1, qty2, qty3, qty4;
private JButton buyButton;
public ShoppingApp() {
setTitle("Shopping App");
setLayout(new GridLayout(6, 2));
title = new JLabel("Welcome to Shopping App");
title.setFont(new Font("Serif", Font.BOLD, 20));
add(title);
item1 = new JLabel("Item 1 (Price $10):");
add(item1);
qty1 = new JTextField();
add(qty1);
item2 = new JLabel("Item 2 (Price $15):");
add(item2);
qty2 = new JTextField();
add(qty2);
item3 = new JLabel("Item 3 (Price $20):");
add(item3);
qty3 = new JTextField();
add(qty3);
item4 = new JLabel("Item 4 (Price $25):");
add(item4);
qty4 = new JTextField();
add(qty4);
buyButton = new JButton("Buy");
add(buyButton);
buyButton.addActionListener(this);
totalPrice = new JLabel();
add(totalPrice);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
int total = 0;
int item1Qty = Integer.parseInt(qty1.getText());
int item2Qty = Integer.parseInt(qty2.getText());
int item3Qty = Integer.parseInt(qty3.getText());
int item4Qty = Integer.parseInt(qty4.getText());
total += item1Qty * 10;
total += item2Qty * 15;
total += item3Qty * 20;
total += item4Qty * 25;
totalPrice.setText("Total Price: $" + total);
}
public static void main(String[] args) {
new ShoppingApp();
}
}
```
这个程序具有一个简单的 GUI 界面,它包括四个商品和购买按钮。当用户点击购买按钮时,程序将计算所有商品的数量和总价,并将其显示在标签上。
阅读全文
相关推荐
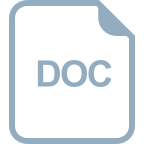
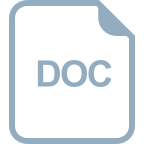
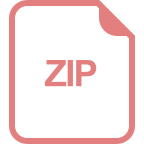
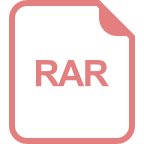
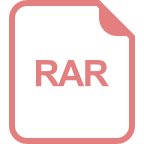
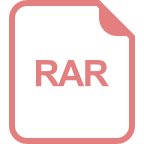
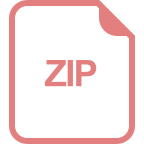
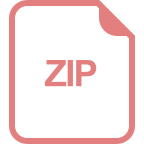
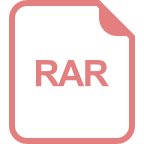
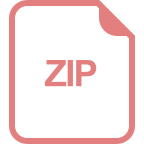
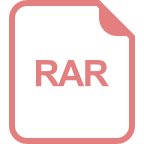
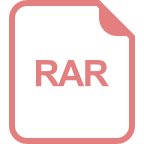
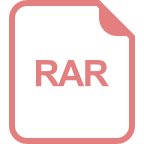
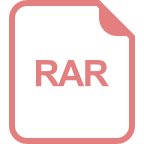
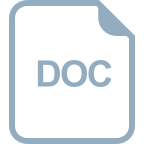
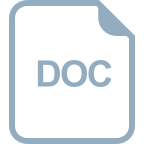
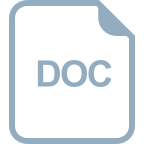
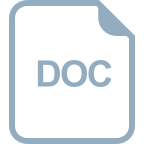