本实验的目的是让学生掌握Java多线程机制,学会两种不同的线程的创建方法。 要求: 编写程序:创建10个线程,第1个线程计算从1加到10,第2个线程计算11加到20,...,第10个线程计算91加到100,最后把10个线程结果相加并输出。要求尽量能看到他们交叉输出。要求使用两种方法创建线程:Thread子类创建线程和Thread类直接创建线程对象。
时间: 2024-02-17 09:04:37 浏览: 148
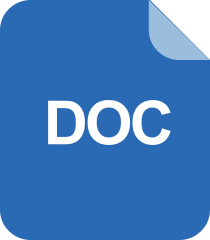
在Java中创建线程有两种方法

好的,以下是Java代码实现:
使用Thread子类创建线程的代码如下:
```java
public class MyThread extends Thread {
private int startNum;
private int endNum;
public int result;
public MyThread(int startNum, int endNum) {
this.startNum = startNum;
this.endNum = endNum;
this.result = 0;
}
@Override
public void run() {
for (int i = startNum; i <= endNum; i++) {
result += i;
}
}
}
public class Main {
public static void main(String[] args) {
MyThread[] threads = new MyThread[10];
for (int i = 0; i < threads.length; i++) {
threads[i] = new MyThread(i * 10 + 1, (i + 1) * 10);
threads[i].start();
}
try {
for (MyThread thread : threads) {
thread.join();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
int result = 0;
for (MyThread thread : threads) {
result += thread.result;
}
System.out.println(result);
}
}
```
使用Thread类直接创建线程对象的代码如下:
```java
public class Main {
public static void main(String[] args) {
Thread[] threads = new Thread[10];
int[] results = new int[10];
for (int i = 0; i < threads.length; i++) {
final int startNum = i * 10 + 1;
final int endNum = (i + 1) * 10;
threads[i] = new Thread(() -> {
for (int j = startNum; j <= endNum; j++) {
results[i] += j;
}
});
threads[i].start();
}
try {
for (Thread thread : threads) {
thread.join();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
int result = 0;
for (int r : results) {
result += r;
}
System.out.println(result);
}
}
```
其中,第二种方法使用了Java 8的Lambda表达式,它能够更简洁地表达一个匿名函数。
阅读全文
相关推荐
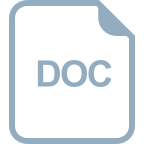
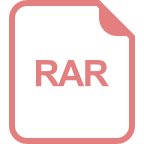
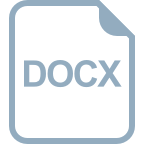
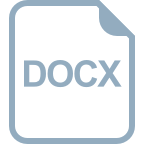
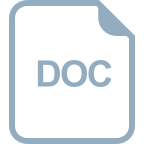
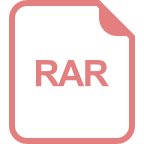
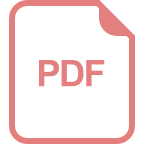
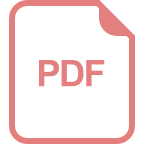
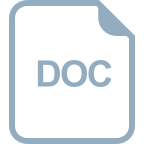
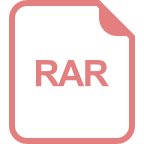
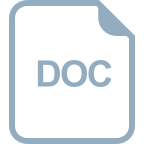
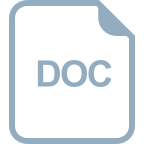
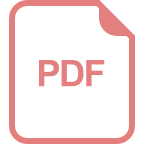
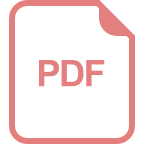
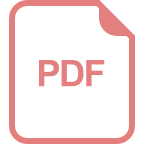
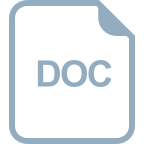
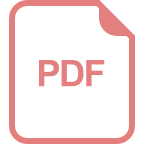